- Python Basics
- Interview Questions
- Python Quiz
- Popular Packages
- Python Projects
- Practice Python
- AI With Python
- Learn Python3
- Python Automation
- Python Web Dev
- DSA with Python
- Python OOPs
- Dictionaries

How to Fix - UnboundLocalError: Local variable Referenced Before Assignment in Python
Developers often encounter the UnboundLocalError Local Variable Referenced Before Assignment error in Python. In this article, we will see what is local variable referenced before assignment error in Python and how to fix it by using different approaches.
What is UnboundLocalError: Local variable Referenced Before Assignment?
This error occurs when a local variable is referenced before it has been assigned a value within a function or method. This error typically surfaces when utilizing try-except blocks to handle exceptions, creating a puzzle for developers trying to comprehend its origins and find a solution.
Below, are the reasons by which UnboundLocalError: Local variable Referenced Before Assignment error occurs in Python :
Nested Function Variable Access
Global variable modification.
In this code, the outer_function defines a variable 'x' and a nested inner_function attempts to access it, but encounters an UnboundLocalError due to a local 'x' being defined later in the inner_function.
In this code, the function example_function tries to increment the global variable 'x', but encounters an UnboundLocalError since it's treated as a local variable due to the assignment operation within the function.
Solution for Local variable Referenced Before Assignment in Python
Below, are the approaches to solve “Local variable Referenced Before Assignment”.
In this code, example_function successfully modifies the global variable 'x' by declaring it as global within the function, incrementing its value by 1, and then printing the updated value.
In this code, the outer_function defines a local variable 'x', and the inner_function accesses and modifies it as a nonlocal variable, allowing changes to the outer function's scope from within the inner function.
Similar Reads
- How to Fix - UnboundLocalError: Local variable Referenced Before Assignment in Python Developers often encounter the UnboundLocalError Local Variable Referenced Before Assignment error in Python. In this article, we will see what is local variable referenced before assignment error in Python and how to fix it by using different approaches. What is UnboundLocalError: Local variable Re 3 min read
- UnboundLocalError Local variable Referenced Before Assignment in Python Handling errors is an integral part of writing robust and reliable Python code. One common stumbling block that developers often encounter is the "UnboundLocalError" raised within a try-except block. This error can be perplexing for those unfamiliar with its nuances but fear not – in this article, w 4 min read
- How to Access Dictionary Values in Python Using For Loop A dictionary is a built-in data type in Python designed to store key-value pairs of data. The most common method to access values in Python is through the use of a for loop. This article explores various approaches to accessing values in a dictionary using a for loop. Access Dictionary Values in Pyt 2 min read
- How to fix "SyntaxError: invalid character" in Python In this article, we will understand the SyntaxError: invalid character in Python through examples, and we will also explore potential approaches to resolve this issue. What is "SyntaxError: invalid character" in Python?Python SyntaxError: Invalid Character occurs when the interpreter encounters a ch 3 min read
- Python | Accessing variable value from code scope Sometimes, we just need to access a variable other than the usual way of accessing by it's name. There are many method by which a variable can be accessed from the code scope. These are by default dictionaries that are created and which keep the variable values as dictionary key-value pair. Let's ta 3 min read
- How To Fix - Python RuntimeWarning: overflow encountered in scalar One such error that developers may encounter is the "Python RuntimeWarning: Overflow Encountered In Scalars". In Python, numeric operations can sometimes trigger a "RuntimeWarning: overflow encountered in a scalar." In this article, we will see what is Python "Python Runtimewarning: Overflow Encount 3 min read
- Different Forms of Assignment Statements in Python We use Python assignment statements to assign objects to names. The target of an assignment statement is written on the left side of the equal sign (=), and the object on the right can be an arbitrary expression that computes an object. There are some important properties of assignment in Python :- 3 min read
- Python Program to Find and Print Address of Variable In this article, we are going to see how to find and print the address of the Python variable. It can be done in these ways: Using id() functionUsing addressof() functionUsing hex() functionMethod 1: Find and Print Address of Variable using id()We can get an address using id() function, id() functio 2 min read
- Accessing Python Function Variable Outside the Function In Python, variables defined within a function have local scope by default. But to Access function variables outside the function usually requires the use of the global keyword, but can we do it without using Global. In this article, we will see how to access a function variable outside the function 4 min read
- Variables under the hood in Python In simple terms, variables are names attached to particular objects in Python. To create a variable, you just need to assign a value and then start using it. The assignment is done with a single equals sign (=): C/C++ Code # Variable named age age = 20 print(age) # Variable named id_number id_no = 4 3 min read
- How to Change Class Attributes By Reference in Python We have the problem of how to change class attributes by reference in Python, we will see in this article how can we change the class attributes by reference in Python. What is Class Attributes?Class attributes are typically defined outside of any method within a class and are shared among all insta 3 min read
- Python program to find number of local variables in a function Given a Python program, task is to find the number of local variables present in a function. Examples: Input : a = 1 b = 2.1 str = 'GeeksForGeeks' Output : 3 We can use the co_nlocals() function which returns the number of local variables used by the function to get the desired result. Code #1: # Im 1 min read
- Python program to create dynamically named variables from user input Given a string input, our task is to write a Python program to create a variable from that input (as a variable name) and assign it to some value. Below are the methods to create dynamically named variables from user input. Using globals() method to create dynamically named variables Here we are usi 2 min read
- Python TabError: Inconsistent Use of Tabs and Spaces in Indentation Python, known for its readability and simplicity, enforces strict indentation rules to structure code. However, encountering a TabError can be frustrating, especially when the code appears to be properly aligned. In this article, we'll explore what a TabError is, and how to resolve TabError in Pytho 2 min read
- Insert a Variable into a String - Python In Python, inserting a variable inside a string is a common task. There are different ways to do this depending on which method you find easiest. Let’s look at some simple methods to insert variables into strings. Using + OperatorThe simplest way to insert a variable into a string is by using the + 1 min read
- Python | Using variable outside and inside the class and method In Python, we can define the variable outside the class, inside the class, and even inside the methods. Let's see, how to use and access these variables throughout the program. Variable defined outside the class: The variables that are defined outside the class can be accessed by any class or any me 3 min read
- SyntaxError: ‘return’ outside function in Python We are given a problem of how to solve the 'Return Outside Function' Error in Python. In this article, we will see how to solve the 'Return Outside Function' Error in Python with reasons for it's occurring and also approaches to solve SyntaxError: ‘return’ outside function” in Python. What is Syntax 4 min read
- Run One Python Script From Another in Python In Python, we can run one file from another using the import statement for integrating functions or modules, exec() function for dynamic code execution, subprocess module for running a script as a separate process, or os.system() function for executing a command to run another Python file within the 5 min read
- Python | Scope resolution when a function is called or defined Python resolves the scope of the parameters of a function in two ways: When the function is definedWhen the function is called When the function is defined Consider this sample program which has a function adder(a, b) which adds an element a to the list b and returns the list b. The default value of 3 min read
- Python Programs
- Python Errors
- Python How-to-fix
Improve your Coding Skills with Practice
What kind of Experience do you want to share?
Fix "local variable referenced before assignment" in Python
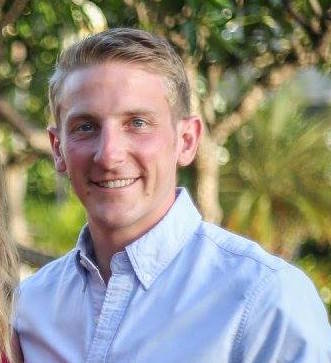
Introduction
If you're a Python developer, you've probably come across a variety of errors, like the "local variable referenced before assignment" error. This error can be a bit puzzling, especially for beginners and when it involves local/global variables.
Today, we'll explain this error, understand why it occurs, and see how you can fix it.
The "local variable referenced before assignment" Error
The "local variable referenced before assignment" error in Python is a common error that occurs when a local variable is referenced before it has been assigned a value. This error is a type of UnboundLocalError , which is raised when a local variable is referenced before it has been assigned in the local scope.
Here's a simple example:
Running this code will throw the "local variable 'x' referenced before assignment" error. This is because the variable x is referenced in the print(x) statement before it is assigned a value in the local scope of the foo function.
Even more confusing is when it involves global variables. For example, the following code also produces the error:
But wait, why does this also produce the error? Isn't x assigned before it's used in the say_hello function? The problem here is that x is a global variable when assigned "Hello ". However, in the say_hello function, it's a different local variable, which has not yet been assigned.
We'll see later in this Byte how you can fix these cases as well.
Fixing the Error: Initialization
One way to fix this error is to initialize the variable before using it. This ensures that the variable exists in the local scope before it is referenced.
Let's correct the error from our first example:
In this revised code, we initialize x with a value of 1 before printing it. Now, when you run the function, it will print 1 without any errors.
Fixing the Error: Global Keyword
Another way to fix this error, depending on your specific scenario, is by using the global keyword. This is especially useful when you want to use a global variable inside a function.
No spam ever. Unsubscribe anytime. Read our Privacy Policy.
Here's how:
In this snippet, we declare x as a global variable inside the function foo . This tells Python to look for x in the global scope, not the local one . Now, when you run the function, it will increment the global x by 1 and print 1 .
Similar Error: NameError
An error that's similar to the "local variable referenced before assignment" error is the NameError . This is raised when you try to use a variable or a function name that has not been defined yet.
Running this code will result in a NameError :
In this case, we're trying to print the value of y , but y has not been defined anywhere in the code. Hence, Python raises a NameError . This is similar in that we are trying to use an uninitialized/undefined variable, but the main difference is that we didn't try to initialize y anywhere else in our code.
Variable Scope in Python
Understanding the concept of variable scope can help avoid many common errors in Python, including the main error of interest in this Byte. But what exactly is variable scope?
In Python, variables have two types of scope - global and local. A variable declared inside a function is known as a local variable, while a variable declared outside a function is a global variable.
Consider this example:
In this code, x is a global variable, and y is a local variable. x can be accessed anywhere in the code, but y can only be accessed within my_function . Confusion surrounding this is one of the most common causes for the "variable referenced before assignment" error.
In this Byte, we've taken a look at the "local variable referenced before assignment" error and another similar error, NameError . We also delved into the concept of variable scope in Python, which is an important concept to understand to avoid these errors. If you're seeing one of these errors, check the scope of your variables and make sure they're being assigned before they're being used.
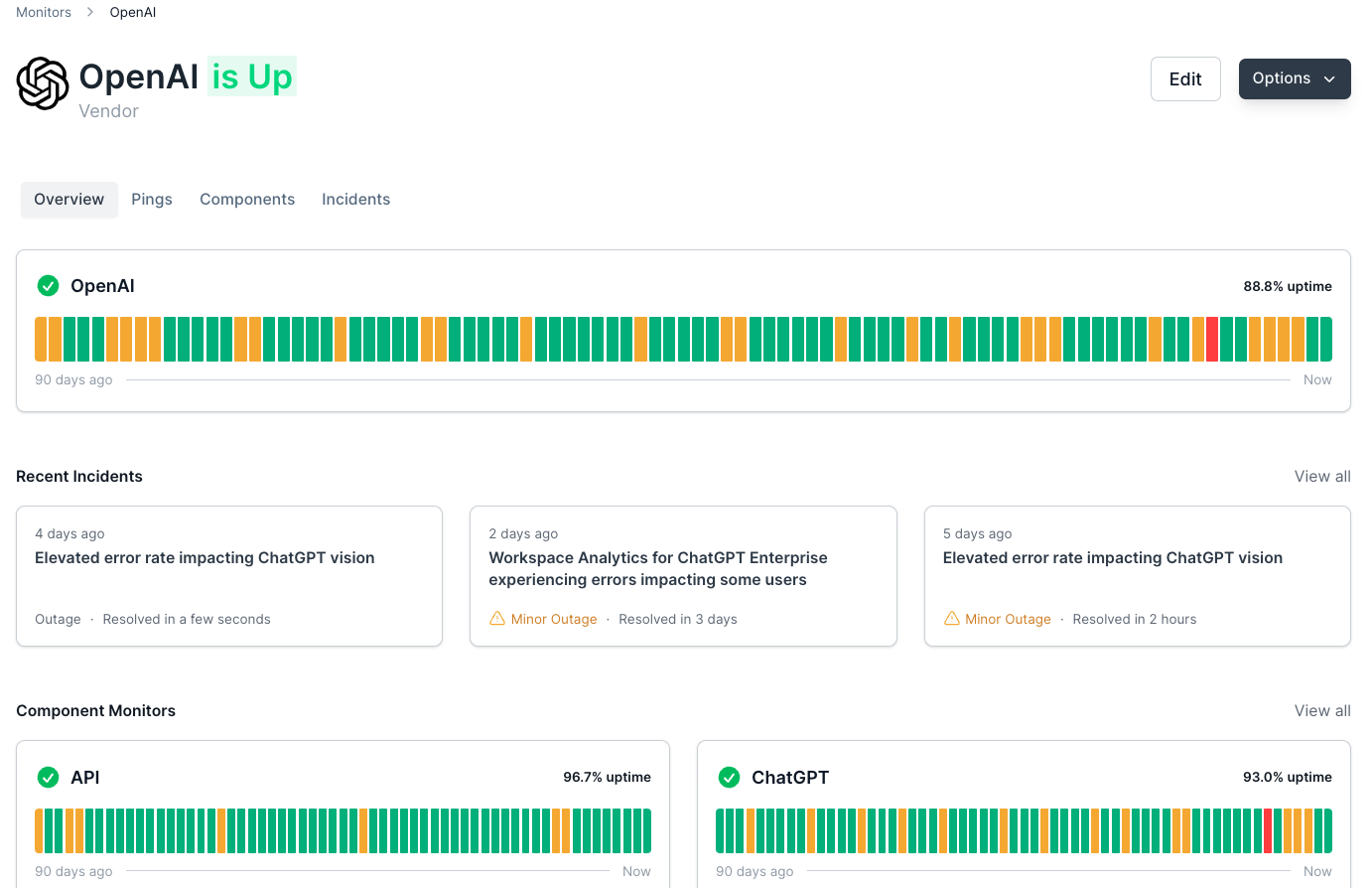
Monitor with Ping Bot
Reliable monitoring for your app, databases, infrastructure, and the vendors they rely on. Ping Bot is a powerful uptime and performance monitoring tool that helps notify you and resolve issues before they affect your customers.
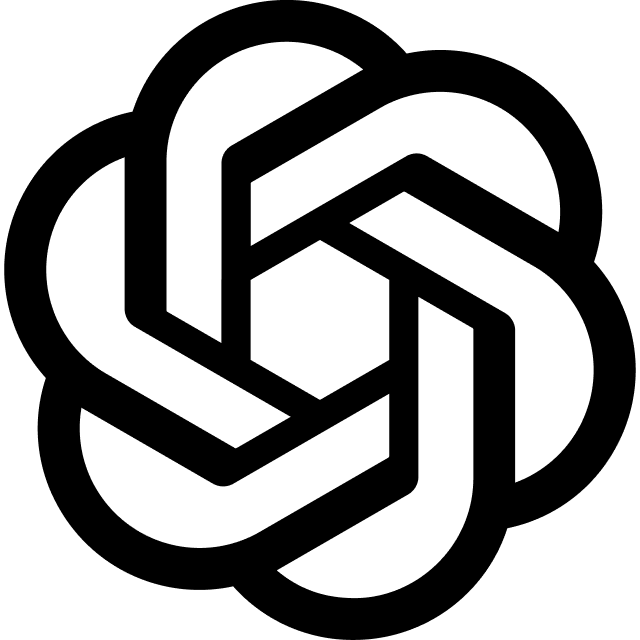
© 2013- 2024 Stack Abuse. All rights reserved.
How to Fix Local Variable Referenced Before Assignment Error in Python
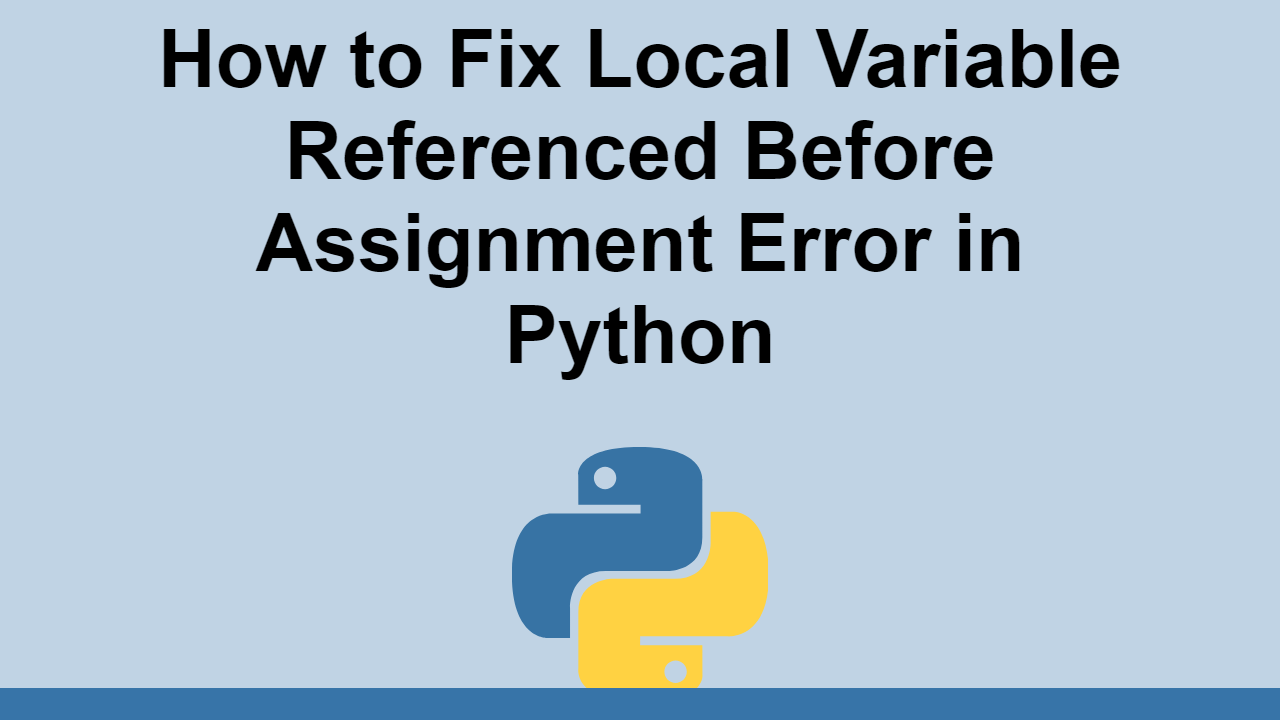
Table of Contents
Fixing local variable referenced before assignment error.
In Python , when you try to reference a variable that hasn't yet been given a value (assigned), it will throw an error.
That error will look like this:
In this post, we'll see examples of what causes this and how to fix it.
Let's begin by looking at an example of this error:
If you run this code, you'll get
The issue is that in this line:
We are defining a local variable called value and then trying to use it before it has been assigned a value, instead of using the variable that we defined in the first line.
If we want to refer the variable that was defined in the first line, we can make use of the global keyword.
The global keyword is used to refer to a variable that is defined outside of a function.
Let's look at how using global can fix our issue here:
Global variables have global scope, so you can referenced them anywhere in your code, thus avoiding the error.
If you run this code, you'll get this output:
In this post, we learned at how to avoid the local variable referenced before assignment error in Python.
The error stems from trying to refer to a variable without an assigned value, so either make use of a global variable using the global keyword, or assign the variable a value before using it.
Thanks for reading!
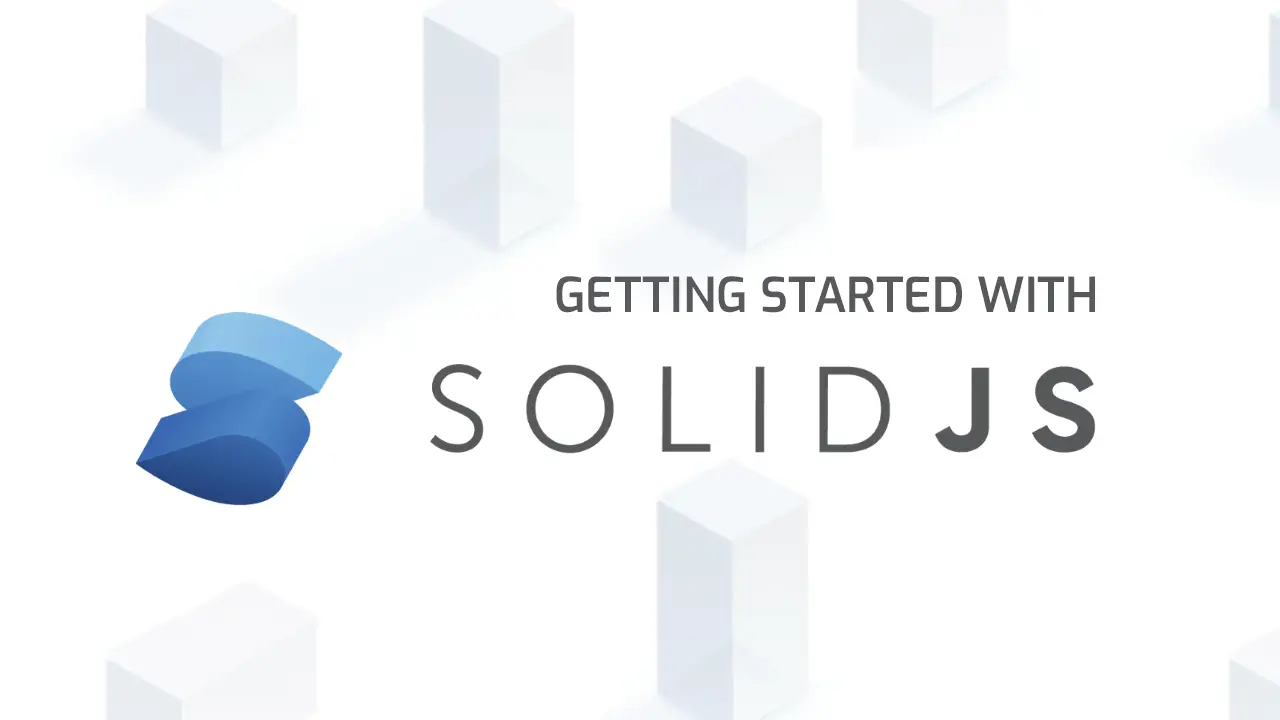
- Privacy Policy
- Terms of Service
Adventures in Machine Learning
4 ways to fix local variable referenced before assignment error in python, resolving the local variable referenced before assignment error in python.
Python is one of the world’s most popular programming languages due to its simplicity, readability, and versatility. Despite its many advantages, when coding in Python, one may encounter various errors, with the most common being the “local variable referenced before assignment” error.
Even the most experienced Python developers have encountered this error at some point in their programming career. In this article, we will look at four effective strategies for resolving the local variable referenced before assignment error in Python.
Strategy 1: Assigning a Value before Referencing
The first strategy is to assign a value to a variable before referencing it. The error occurs when the variable is referenced before it is assigned a value.
This problem can be avoided by initializing the variable before referencing it. For example, let us consider the snippet below:
In the snippet above, the variables x and y are not assigned values before they are referenced in the print statement. Therefore, we will get a local variable “referenced before assignment” error.
To resolve this error, we must initialize the variables before referencing them. We can avoid this error by assigning a value to x and y before they are referenced, as shown below:
Strategy 2: Using the Global Keyword
In Python, variables declared inside a function are considered local variables. Thus, they are separate from other variables declared outside of the function.
If we want to use a variable outside of the function, we must use the global keyword. Using the global keyword tells Python that you want to use the variable that was defined globally, not locally.
For example:
In the code snippet above, the global keyword tells Python to use the variable x defined outside of the function rather than a local variable named x . Thus, Python will output 30.
Strategy 3: Adding Input Parameters for Functions
Another way to avoid the local variable referenced before assignment error is by adding input parameters to functions.
In the code snippet above, x and y are variables that are passed into the add_numbers function as arguments.
This approach allows us to avoid the local variable referenced before assignment error because the variables are being passed into the function as input parameters.
Strategy 4: Initializing Variables before Loops or Conditionals
Finally, it’s also a good practice to initialize the variables before loops or conditionals.
If you are defining a variable within a loop, you must initialize it before the loop starts. This way, the variable already exists, and we can update the value inside the loop.
In the code snippet above, the variable sum has been initialized with the value of 0 before the loop runs. Thus, we can update and use the variable inside the loop.
In conclusion, the “local variable referenced before assignment” error is a common issue in Python. However, with the strategies discussed in this article, you can avoid the error and write clean Python code.
Remember to initialize your variables, use the global keyword, add input parameters in functions, and initialize variables before loops or conditionals. By following these techniques, your Python code will be error-free and much easier to manage.
In essence, this article has provided four key strategies for resolving the “local variable referenced before assignment” error that is common in Python. These strategies include initializing variables before referencing, using the global keyword, adding input parameters to functions, and initializing variables before loops or conditionals.
These techniques help to ensure clean code that is free from errors. By implementing these strategies, developers can improve their code quality and avoid time-wasting errors that can occur in their work.
Popular Posts
Mastering numpy indexing: how to solve indexerror and more, mastering pandas: exploring the power of groupby() function, solving the ‘tuple’ object not callable error in python programming.
- Terms & Conditions
- Privacy Policy
How to fix UnboundLocalError: local variable 'x' referenced before assignment in Python
by Nathan Sebhastian
Posted on May 26, 2023
Reading time: 2 minutes
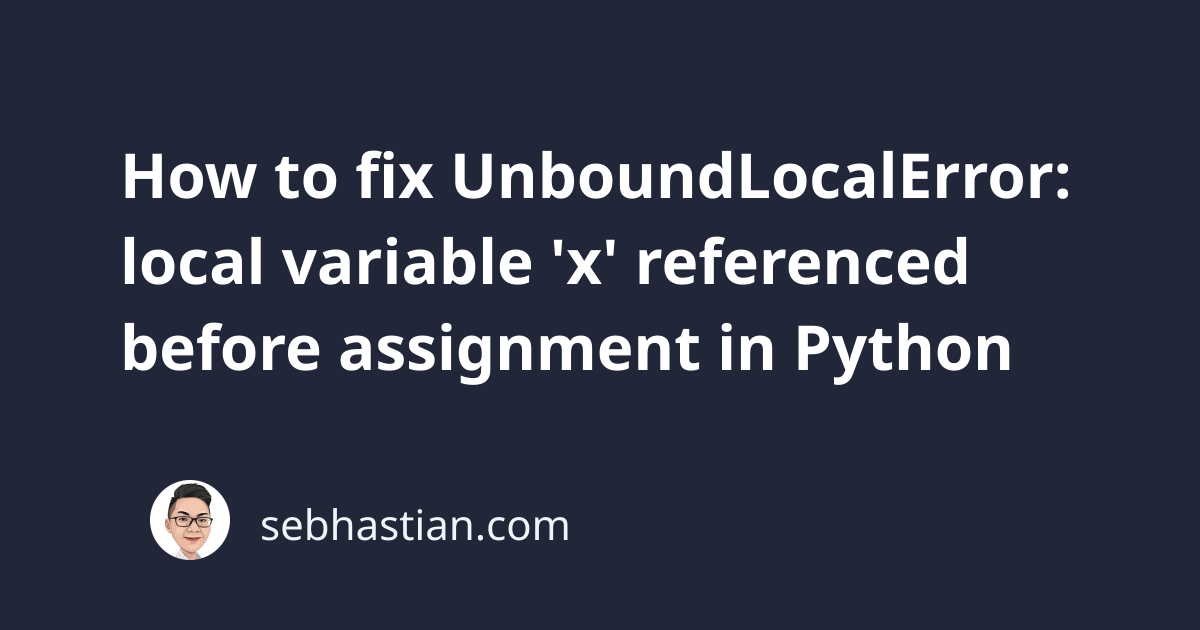
One error you might encounter when running Python code is:
This error commonly occurs when you reference a variable inside a function without first assigning it a value.
You could also see this error when you forget to pass the variable as an argument to your function.
Let me show you an example that causes this error and how I fix it in practice.
How to reproduce this error
Suppose you have a variable called name declared in your Python code as follows:
Next, you created a function that uses the name variable as shown below:
When you execute the code above, you’ll get this error:
This error occurs because you both assign and reference a variable called name inside the function.
Python thinks you’re trying to assign the local variable name to name , which is not the case here because the original name variable we declared is a global variable.
How to fix this error
To resolve this error, you can change the variable’s name inside the function to something else. For example, name_with_title should work:
As an alternative, you can specify a name parameter in the greet() function to indicate that you require a variable to be passed to the function.
When calling the function, you need to pass a variable as follows:
This code allows Python to know that you intend to use the name variable which is passed as an argument to the function as part of the newly declared name variable.
Still, I would say that you need to use a different name when declaring a variable inside the function. Using the same name might confuse you in the future.
Here’s the best solution to the error:
Now it’s clear that we’re using the name variable given to the function as part of the value assigned to name_with_title . Way to go!
The UnboundLocalError: local variable 'x' referenced before assignment occurs when you reference a variable inside a function before declaring that variable.
To resolve this error, you need to use a different variable name when referencing the existing variable, or you can also specify a parameter for the function.
I hope this tutorial is useful. See you in other tutorials.
Take your skills to the next level ⚡️
I'm sending out an occasional email with the latest tutorials on programming, web development, and statistics. Drop your email in the box below and I'll send new stuff straight into your inbox!
Hello! This website is dedicated to help you learn tech and data science skills with its step-by-step, beginner-friendly tutorials. Learn statistics, JavaScript and other programming languages using clear examples written for people.
Learn more about this website
Connect with me on Twitter
Or LinkedIn
Type the keyword below and hit enter
Click to see all tutorials tagged with:
Consultancy
- Technology Consulting
- Customer Experience Consulting
- Solution Architect Consulting

Software Development Services
- Ecommerce Development
- Web App Development
- Mobile App Development
- SAAS Product Development
- Content Management System
- System Integration & Data Migration
- Cloud Computing
- Computer Vision
Dedicated Development Team
- Full Stack Developers For Hire
- Offshore Development Center
Marketing & Creative Design
- UX/UI Design
- Customer Experience Optimization
- Digital Marketing
- Devops Services
- Service Level Management
- Security Services
- Odoo gold partner
By Industry
- Retail & Ecommerce
- Manufacturing
- Import & Distribution
- Financical & Banking
- Technology For Startups
Business Model
- MARKETPLACE ECOMMERCE
Our realized projects
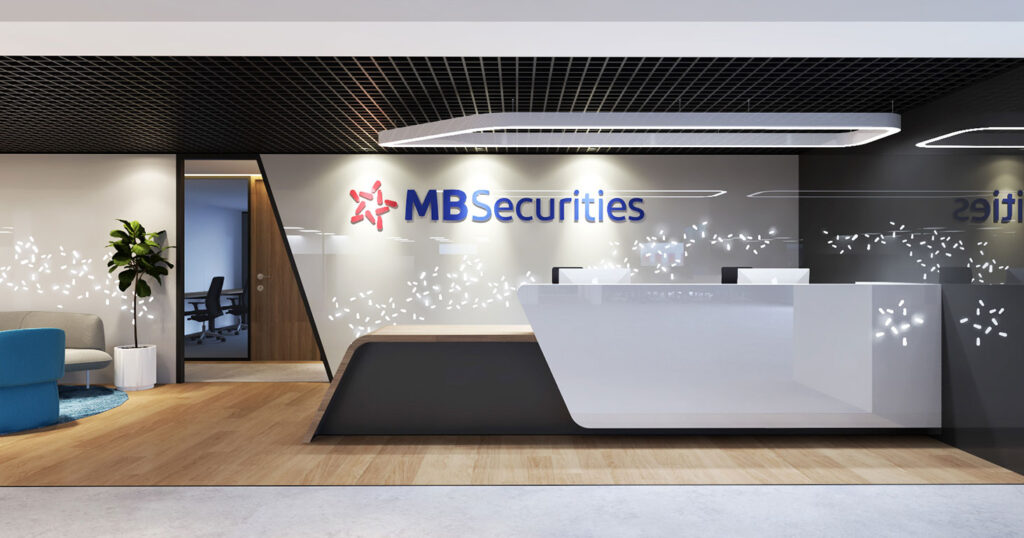
MB Securities - A Premier Brokerage
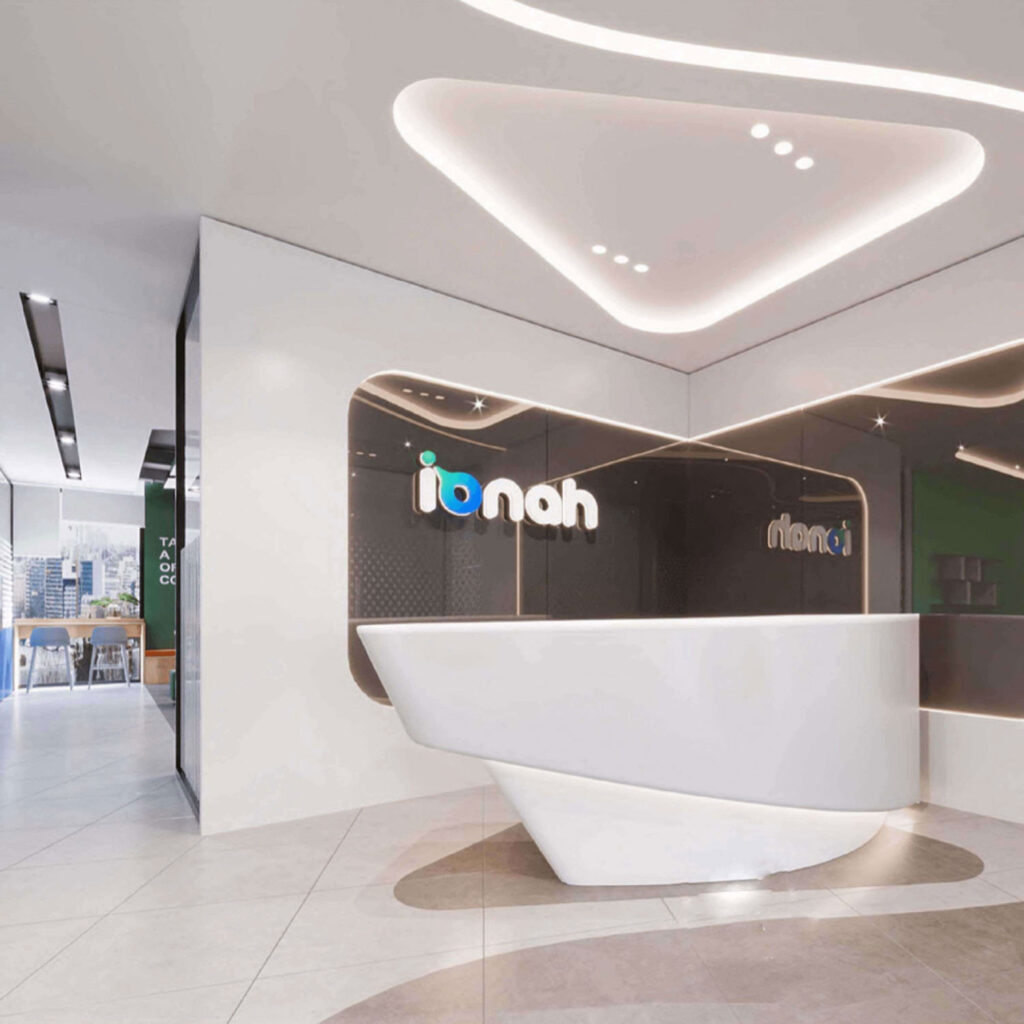
iONAH - A Pioneer in Consumer Electronics Industry
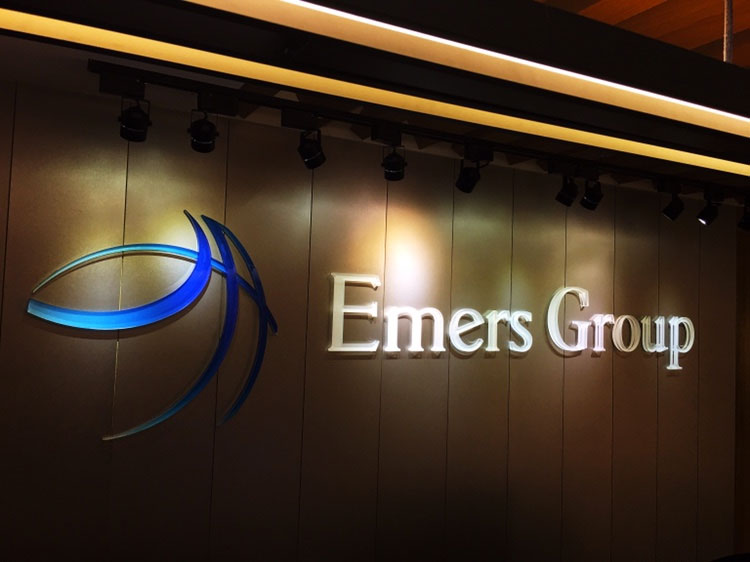
Emers Group - An Official Nike Distributing Agent
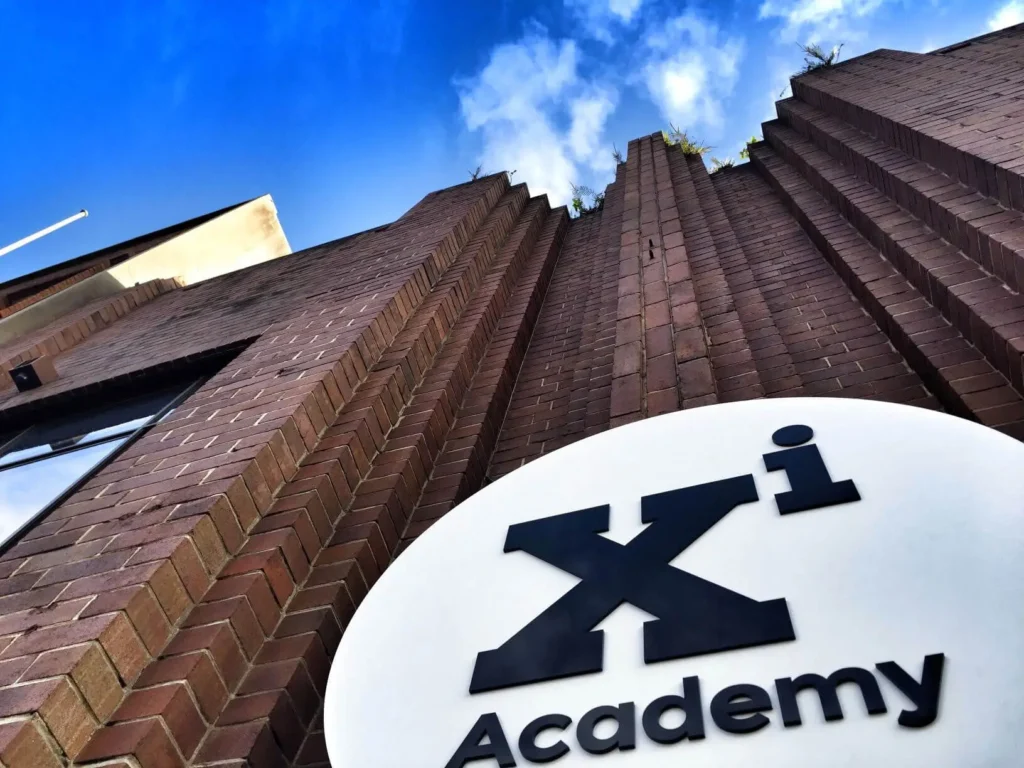
Academy Xi - An Australian-based EdTech Startup
- Market insight
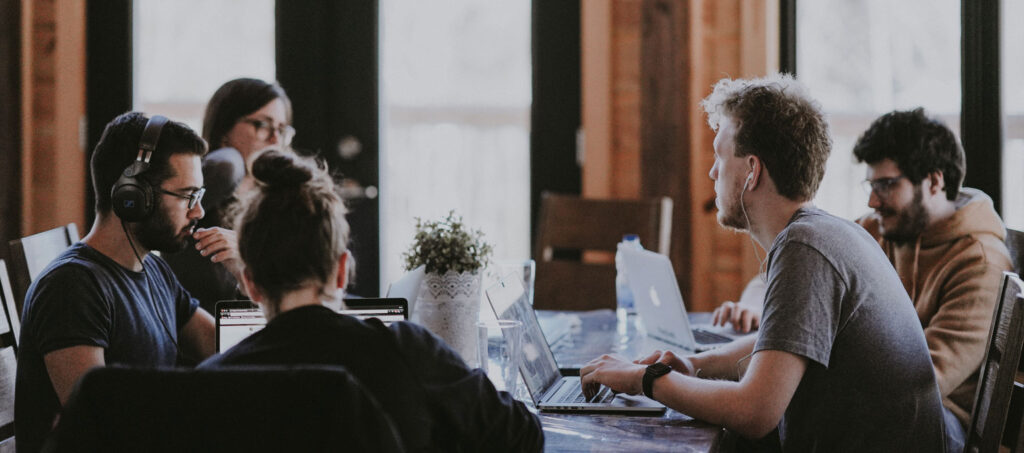
- Ohio Digital
- Onnet Consoulting
What is UnboundLocalError: local variable referenced before assignment?
Trying to assign a value to a variable that does not have local scope can result in this error:
Python has a simple rule to determine the scope of a variable. To clarify, a variable is assigned in a function, that variable is local. Because it is assumed that when you define a variable inside a function, you only need to access it inside that function.
There are two variable scopes in Python: local and global. Global variables are accessible throughout an entire program. Whereas, local variables are only accessible within the function in which they are originally defined.
An example of Local variable referenced before assignment
We’re going to write a program that calculates the grade a student has earned in class.
Firstly, we start by declaring two variables:
These variables store the numerical and letter grades a student has earned, respectively. By default, the value of “letter” is “F”. Then, we write a function that calculates a student’s letter grade based on their numerical grade using an “if” statement:
Finally, we call our function:
This line of code prints out the value returned by the calculate_grade() function to the console. We pass through one parameter into our function: numerical. This is the numerical value of the grade a student has earned.
Let’s run our code of Local variable referenced before assignment and see what happens:
Here is an error!
The Solution of Local variable referenced before assignment
The code returns an error: Unboundlocalerror local variable referenced before assignment because we reference “letter” before we assign it.
We have set the value of “numerical” to 42. Our if statement does not set a value for any grade over 50. This means that when we call our calculate_grade() function, our return statement does not know the value to which we are referring.
Moreover, we do define “letter” at the start of our program. However, we define it in the global context. Because Python treats “return letter” as trying to return a local variable called “letter”, not a global variable.
Therefore, this problem of variable referenced before assignment could be solved in two ways. Firstly, we can add an else statement to our code. This ensures we declare “letter” before we try to return it:
Let’s try to run our code again:
Our code successfully prints out the student’s grade. This approach is good because it lets us keep “letter” in the local context. To clarify, we could even remove the “letter = “F”” statement from the top of our code because we do not use it in the global context.
Alternatively, we could use the “global” keyword to make our global keyword available in the local context in our calculate_grade() function:
We use the “global” keyword at the start of our function.
This keyword changes the scope of our variable to a global variable. This means the “return” statement will no longer treat “letter” like a local variable. Let’s run our code. Our code returns: F.
The code works successfully! Let’s try it using a different grade number by setting the value of “numerical” to a new number:
Our code returns: B.
Finally, we have fixed the local variable referenced before assignment error in the code.
To sum up, as you can see, the UnboundLocalError: local variable referenced before assignment error is raised when you try to assign a value to a local variable before it has been declared. Then, you can solve this error by ensuring that a local variable is declared before you assign it a value. Moreover, if a variable is declared globally that you want to access in a function, you can use the “global” keyword to change its value. In case you have any inquiry, let’s CONTACT US . With a lot of experience in Mobile app development services , we will surely solve it for you instantly.
>>> Read more
- Average python length: How to find it with examples
- List assignment index out of range: Python indexerror solution you should know
- Spyder vs Pycharm: The detailed comparison to get best option for Python programming
- fix python error , Local variable referenced before assignment , python , python dictionary , python error , python learning , UnboundLocalError , UnboundLocalError in Python
Our Other Services
- E-commerce Development
- Web Apps Development
- Web CMS Development
- Mobile Apps Development
- Software Consultant & Development
- System Integration & Data Migration
- Dedicated Developers & Testers For Hire
- Remote Working Team
- Saas Products Development
- Web/Mobile App Development
- Outsourcing
- Hiring Developers
- Digital Transformation
- Advanced SEO Tips
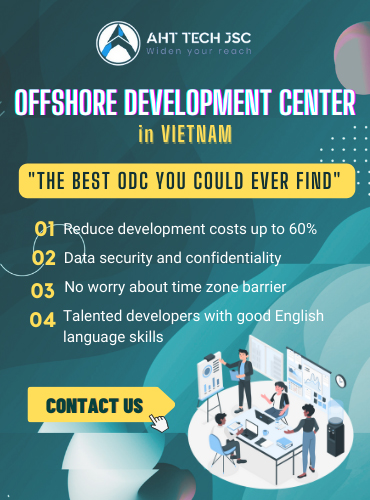
Lastest News
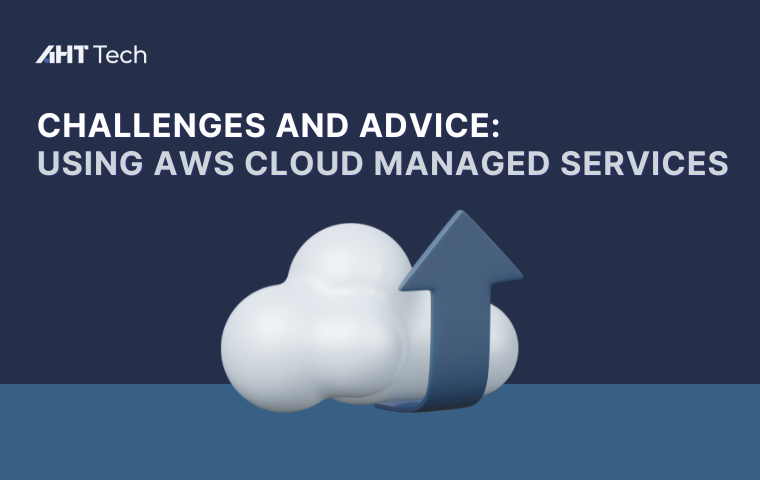
Challenges and Advices When Using AWS Cloud Managed Services
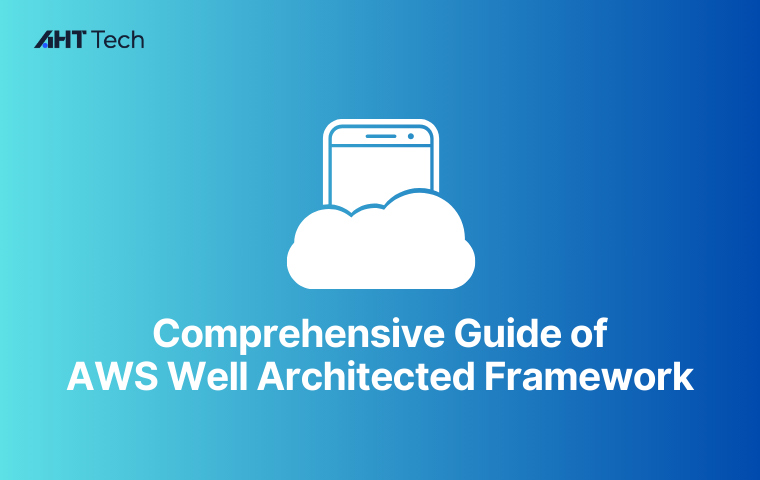
Comprehensive Guide about AWS Well Architected Framework
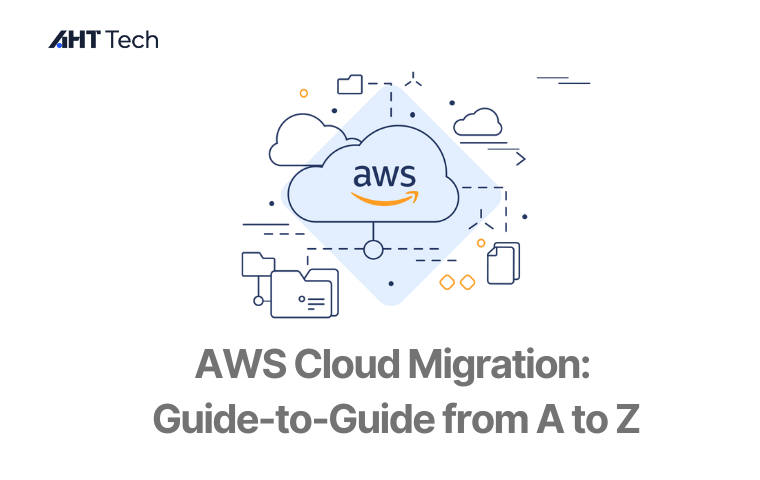
AWS Cloud Migration: Guide-to-Guide from A to Z
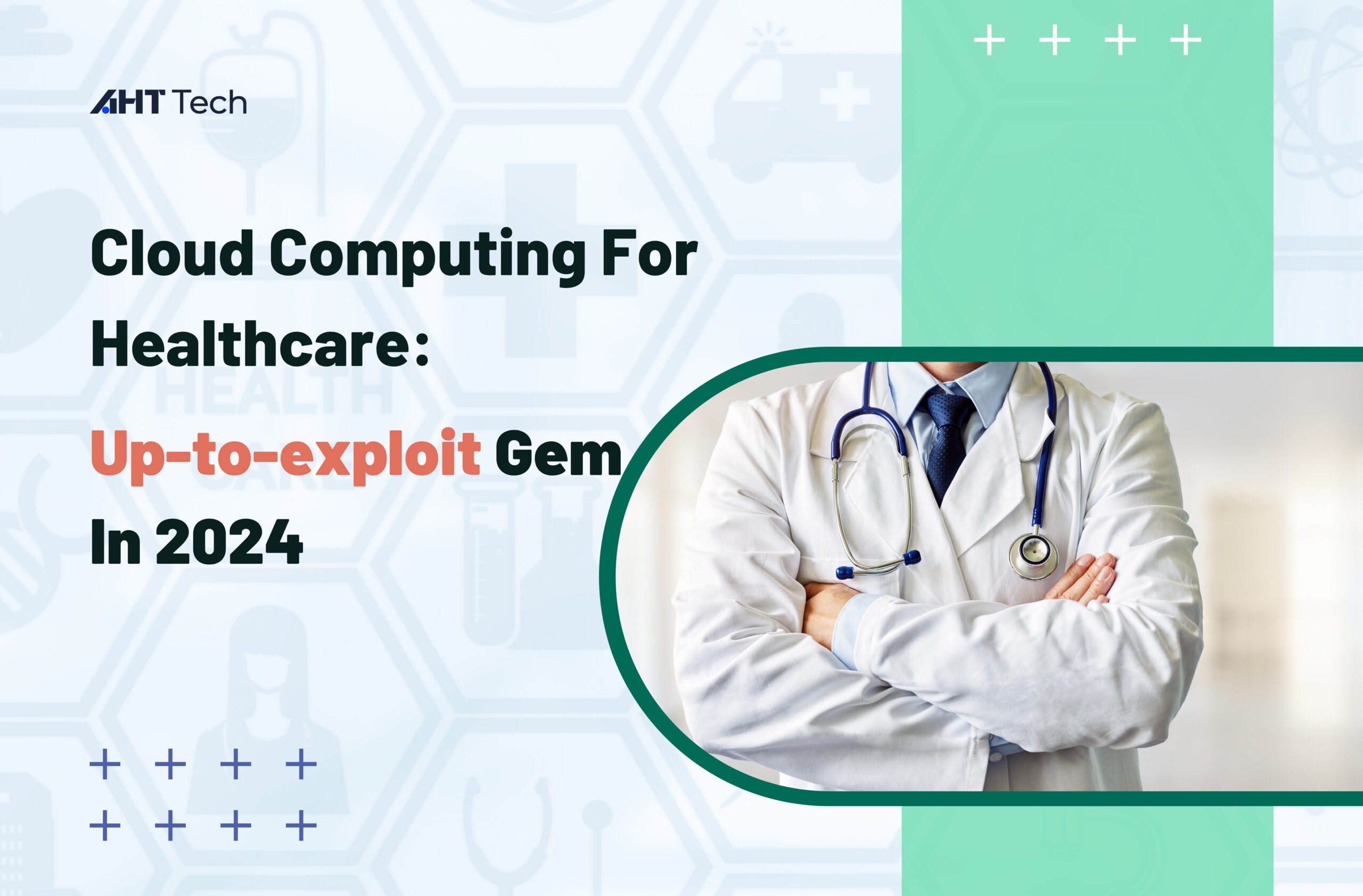
Uncover The Treasures Of Cloud Computing For Healthcare
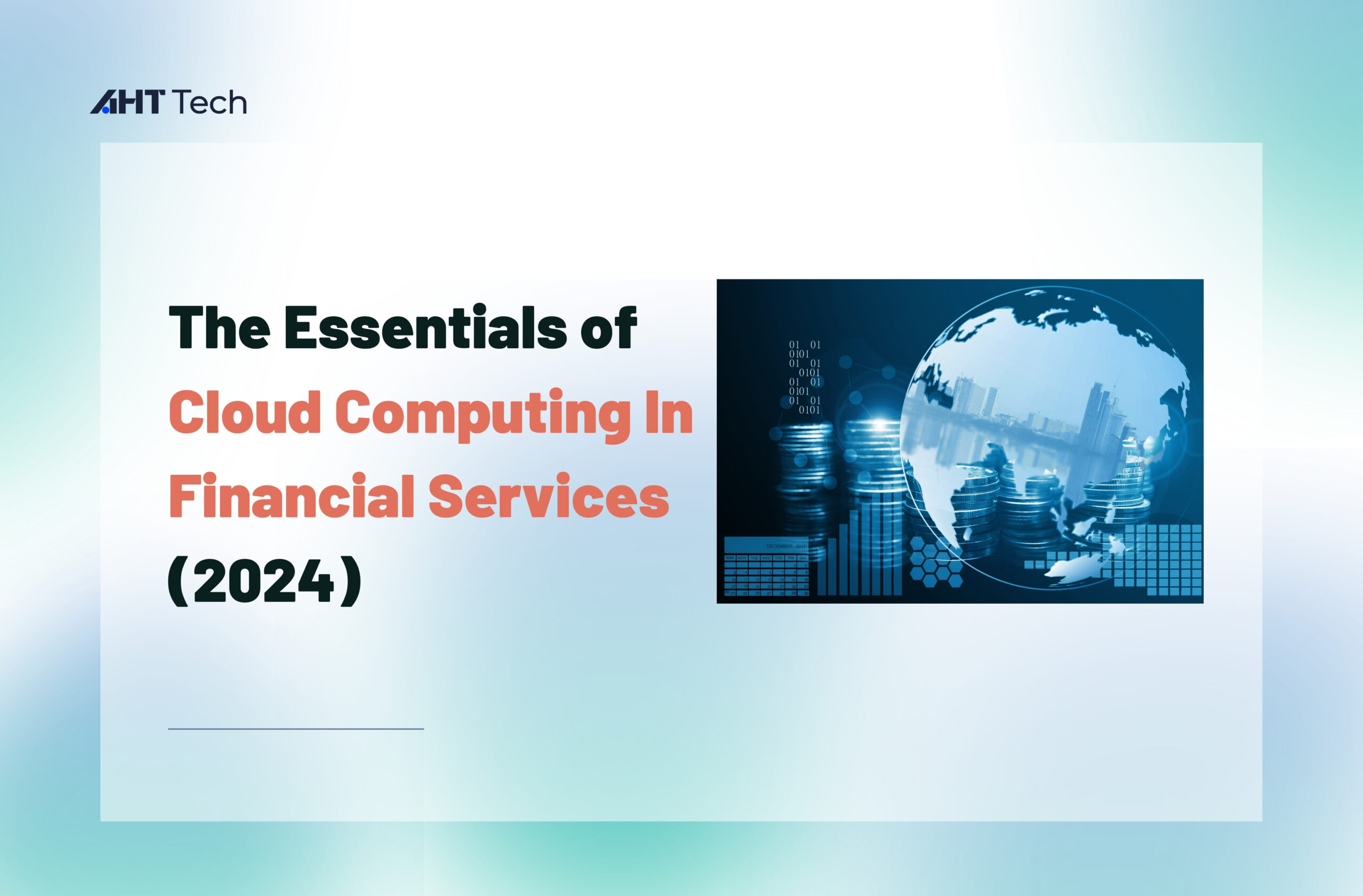
A Synopsis of Cloud Computing in Financial Services
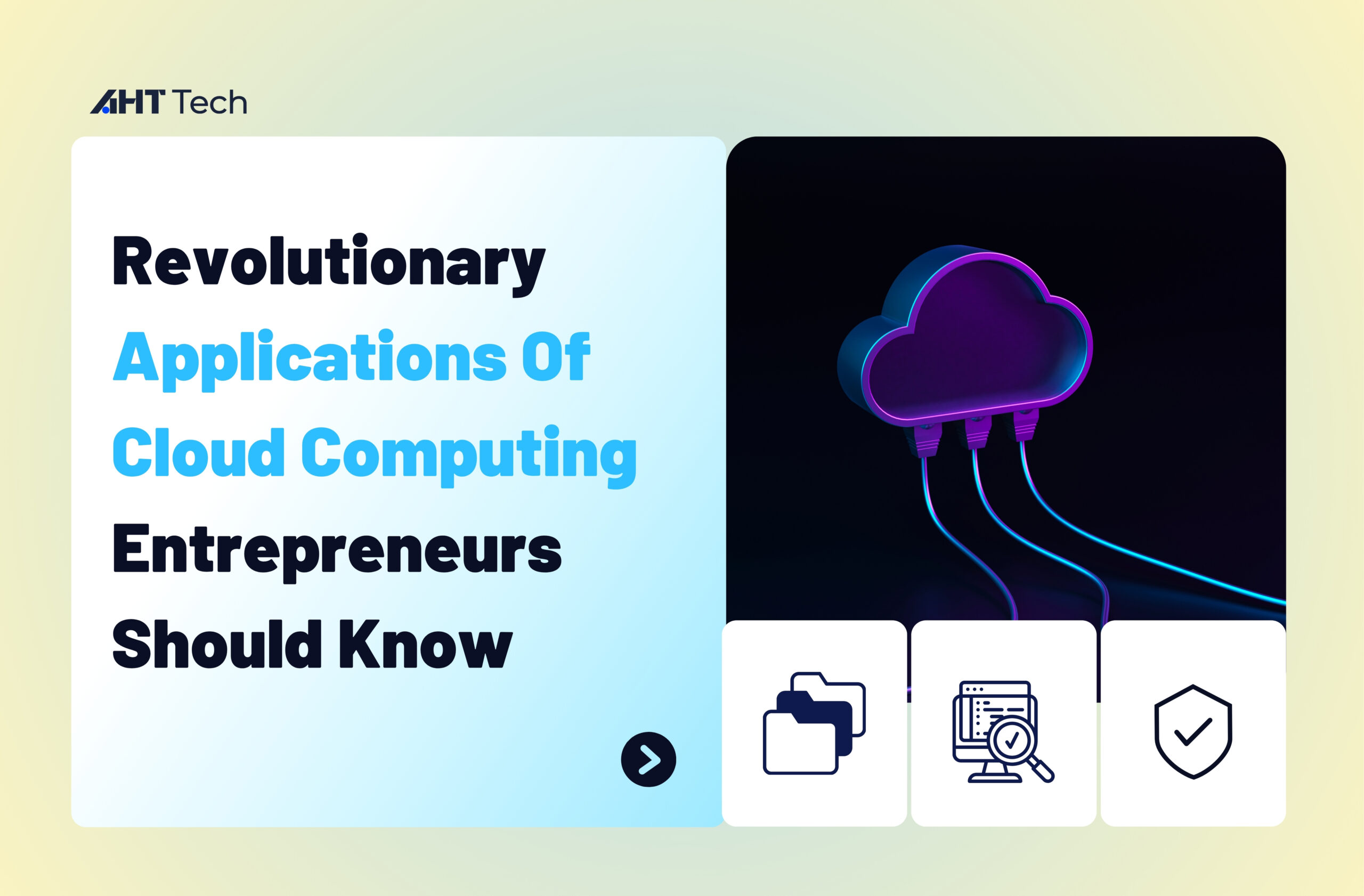
Discover Cutting-Edge Cloud Computing Applications To Optimize Business Resources
Tailor your experience
- Success Stories
Copyright ©2007 – 2021 by AHT TECH JSC. All Rights Reserved.

Thank you for your message. It has been sent.
Explore your training options in 10 minutes Get Started
- Graduate Stories
- Partner Spotlights
- Bootcamp Prep
- Bootcamp Admissions
- University Bootcamps
- Coding Tools
- Software Engineering
- Web Development
- Data Science
- Tech Guides
- Tech Resources
- Career Advice
- Online Learning
- Internships
- Apprenticeships
- Tech Salaries
- Associate Degree
- Bachelor's Degree
- Master's Degree
- University Admissions
- Best Schools
- Certifications
- Bootcamp Financing
- Higher Ed Financing
- Scholarships
- Financial Aid
- Best Coding Bootcamps
- Best Online Bootcamps
- Best Web Design Bootcamps
- Best Data Science Bootcamps
- Best Technology Sales Bootcamps
- Best Data Analytics Bootcamps
- Best Cybersecurity Bootcamps
- Best Digital Marketing Bootcamps
- Los Angeles
- San Francisco
- Browse All Locations
- Digital Marketing
- Machine Learning
- See All Subjects
- Bootcamps 101
- Full-Stack Development
- Career Changes
- View all Career Discussions
- Mobile App Development
- Cybersecurity
- Product Management
- UX/UI Design
- What is a Coding Bootcamp?
- Are Coding Bootcamps Worth It?
- How to Choose a Coding Bootcamp
- Best Online Coding Bootcamps and Courses
- Best Free Bootcamps and Coding Training
- Coding Bootcamp vs. Community College
- Coding Bootcamp vs. Self-Learning
- Bootcamps vs. Certifications: Compared
- What Is a Coding Bootcamp Job Guarantee?
- How to Pay for Coding Bootcamp
- Ultimate Guide to Coding Bootcamp Loans
- Best Coding Bootcamp Scholarships and Grants
- Education Stipends for Coding Bootcamps
- Get Your Coding Bootcamp Sponsored by Your Employer
- GI Bill and Coding Bootcamps
- Tech Intevriews
- Our Enterprise Solution
- Connect With Us
- Publication
- Reskill America
- Partner With Us
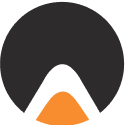
- Resource Center
- Bachelor’s Degree
- Master’s Degree
Python local variable referenced before assignment Solution
When you start introducing functions into your code, you’re bound to encounter an UnboundLocalError at some point. This error is raised when you try to use a variable before it has been assigned in the local context .
In this guide, we talk about what this error means and why it is raised. We walk through an example of this error in action to help you understand how you can solve it.
Find your bootcamp match
What is unboundlocalerror: local variable referenced before assignment.
Trying to assign a value to a variable that does not have local scope can result in this error:
Python has a simple rule to determine the scope of a variable. If a variable is assigned in a function , that variable is local. This is because it is assumed that when you define a variable inside a function you only need to access it inside that function.
There are two variable scopes in Python: local and global. Global variables are accessible throughout an entire program; local variables are only accessible within the function in which they are originally defined.
Let’s take a look at how to solve this error.
An Example Scenario
We’re going to write a program that calculates the grade a student has earned in class.
We start by declaring two variables:
These variables store the numerical and letter grades a student has earned, respectively. By default, the value of “letter” is “F”. Next, we write a function that calculates a student’s letter grade based on their numerical grade using an “if” statement :
Finally, we call our function:
This line of code prints out the value returned by the calculate_grade() function to the console. We pass through one parameter into our function: numerical. This is the numerical value of the grade a student has earned.
Let’s run our code and see what happens:
An error has been raised.
The Solution
Our code returns an error because we reference “letter” before we assign it.
We have set the value of “numerical” to 42. Our if statement does not set a value for any grade over 50. This means that when we call our calculate_grade() function, our return statement does not know the value to which we are referring.
We do define “letter” at the start of our program. However, we define it in the global context. Python treats “return letter” as trying to return a local variable called “letter”, not a global variable.
We solve this problem in two ways. First, we can add an else statement to our code. This ensures we declare “letter” before we try to return it:
Let’s try to run our code again:
Our code successfully prints out the student’s grade.
If you are using an “if” statement where you declare a variable, you should make sure there is an “else” statement in place. This will make sure that even if none of your if statements evaluate to True, you can still set a value for the variable with which you are going to work.
Alternatively, we could use the “global” keyword to make our global keyword available in the local context in our calculate_grade() function. However, this approach is likely to lead to more confusing code and other issues. In general, variables should not be declared using “global” unless absolutely necessary . Your first, and main, port of call should always be to make sure that a variable is correctly defined.
In the example above, for instance, we did not check that the variable “letter” was defined in all use cases.
That’s it! We have fixed the local variable error in our code.
The UnboundLocalError: local variable referenced before assignment error is raised when you try to assign a value to a local variable before it has been declared. You can solve this error by ensuring that a local variable is declared before you assign it a value.
Now you’re ready to solve UnboundLocalError Python errors like a professional developer !
About us: Career Karma is a platform designed to help job seekers find, research, and connect with job training programs to advance their careers. Learn about the CK publication .
What's Next?

Get matched with top bootcamps
Ask a question to our community, take our careers quiz.
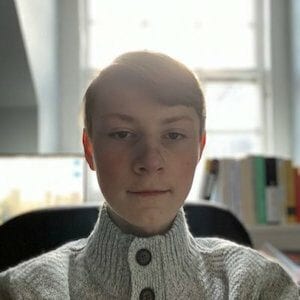
Leave a Reply Cancel reply
Your email address will not be published. Required fields are marked *
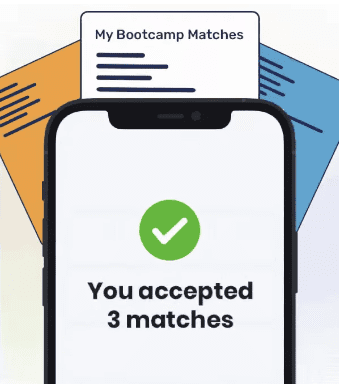

IMAGES
VIDEO
COMMENTS
Why does PyCharm highlight the boolean variable nearby the return with Local variable "boolean" might be referenced before assignment? This code checks whether a number is prime or not: print x. if x < 2: return False. if x == 2: return True. if x == 3: return True. for i in range(2, int(math.sqrt(x))+1): if x % i == 0: boolean = False. break.
In this article, we will see what is local variable referenced before assignment error in Python and how to fix it by using different approaches. What is UnboundLocalError: Local variable Referenced Before Assignment? This error occurs when a local variable is referenced before it has been assigned a value within a function or method.
This error is a type of UnboundLocalError, which is raised when a local variable is referenced before it has been assigned in the local scope. Here's a simple example: def foo (): print (x) x = 1 foo() Running this code will throw the "local variable 'x' referenced before assignment" error.
You're getting a reference before assignment error because the interpreter is ignoring your malformed assignment of the variable data. If you make the two changes above (change to equals sign, add second double quote), it will assign the variable correctly.
In this post, we learned at how to avoid the local variable referenced before assignment error in Python. The error stems from trying to refer to a variable without an assigned value, so either make use of a global variable using the global keyword, or assign the variable a value before using it.
In this article, we will look at four effective strategies for resolving the local variable referenced before assignment error in Python. The first strategy is to assign a value to a variable before referencing it. The error occurs when the variable is referenced before it is assigned a value.
The UnboundLocalError: local variable 'x' referenced before assignment occurs when you reference a variable inside a function before declaring that variable. To resolve this error, you need to use a different variable name when referencing the existing variable, or you can also specify a parameter for the function.
Trying to assign a value to a variable that does not have local scope can result in this error: 1 UnboundLocalError: local variable referenced before assignment. Python has a simple rule to determine the scope of a variable. To clarify, a variable is assigned in a function, that variable is local.
The UnboundLocalError: local variable referenced before assignment error is raised when you try to assign a value to a local variable before it has been declared. You can solve this error by ensuring that a local variable is declared before you assign it a value.
In this video, we tackle a common issue that many Python developers encounter: the 'Local Variable Might Be Referenced Before Assignment' error. This error c...