[SOLVED] Local Variable Referenced Before Assignment
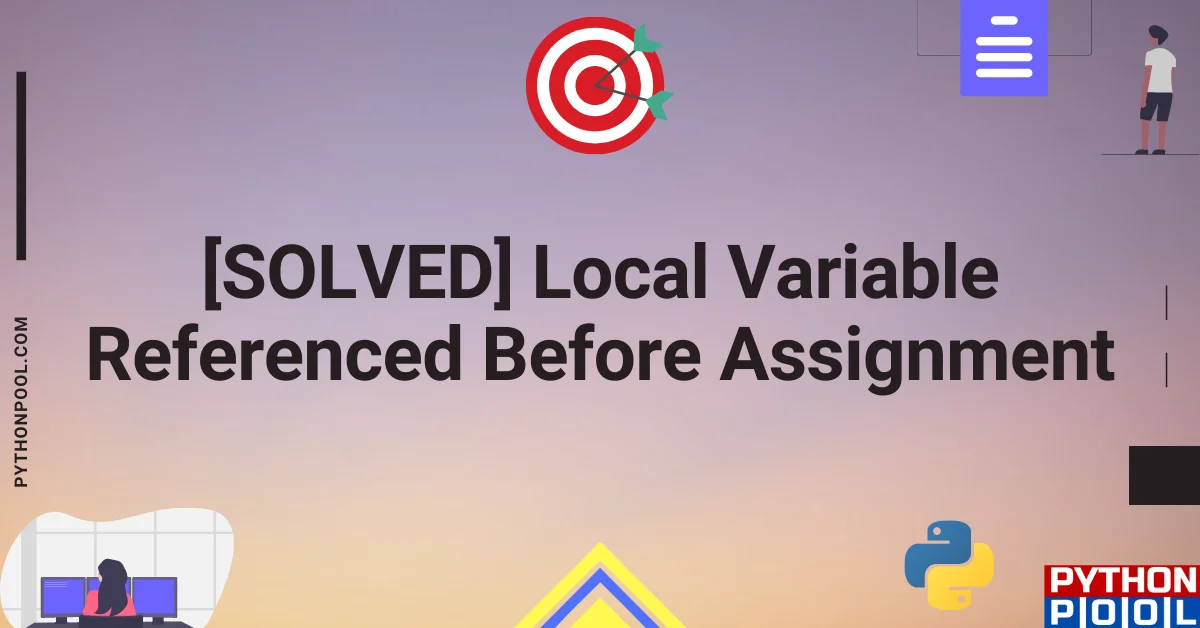
Python treats variables referenced only inside a function as global variables. Any variable assigned to a function’s body is assumed to be a local variable unless explicitly declared as global.

Why Does This Error Occur?
Unboundlocalerror: local variable referenced before assignment occurs when a variable is used before its created. Python does not have the concept of variable declarations. Hence it searches for the variable whenever used. When not found, it throws the error.
Before we hop into the solutions, let’s have a look at what is the global and local variables.
Local Variable Declarations vs. Global Variable Declarations
Local Variables | Global Variables |
---|---|
A variable is declared primarily within a Python function. | Global variables are in the global scope, outside a function. |
A local variable is created when the function is called and destroyed when the execution is finished. | A Variable is created upon execution and exists in memory till the program stops. |
Local Variables can only be accessed within their own function. | All functions of the program can access global variables. |
Local variables are immune to changes in the global scope. Thereby being more secure. | Global Variables are less safer from manipulation as they are accessible in the global scope. |
![local variable 'img_gt' referenced before assignment [Fixed] typeerror can’t compare datetime.datetime to datetime.date](https://www.pythonpool.com/wp-content/uploads/2024/01/typeerror-cant-compare-datetime.datetime-to-datetime.date_-300x157.webp)
Local Variable Referenced Before Assignment Error with Explanation
Try these examples yourself using our Online Compiler.
Let’s look at the following function:

Explanation
The variable myVar has been assigned a value twice. Once before the declaration of myFunction and within myFunction itself.
Using Global Variables
Passing the variable as global allows the function to recognize the variable outside the function.
Create Functions that Take in Parameters
Instead of initializing myVar as a global or local variable, it can be passed to the function as a parameter. This removes the need to create a variable in memory.
UnboundLocalError: local variable ‘DISTRO_NAME’
This error may occur when trying to launch the Anaconda Navigator in Linux Systems.
Upon launching Anaconda Navigator, the opening screen freezes and doesn’t proceed to load.
Try and update your Anaconda Navigator with the following command.
If solution one doesn’t work, you have to edit a file located at
After finding and opening the Python file, make the following changes:
In the function on line 159, simply add the line:
DISTRO_NAME = None
Save the file and re-launch Anaconda Navigator.
DJANGO – Local Variable Referenced Before Assignment [Form]
The program takes information from a form filled out by a user. Accordingly, an email is sent using the information.
Upon running you get the following error:
We have created a class myForm that creates instances of Django forms. It extracts the user’s name, email, and message to be sent.
A function GetContact is created to use the information from the Django form and produce an email. It takes one request parameter. Prior to sending the email, the function verifies the validity of the form. Upon True , .get() function is passed to fetch the name, email, and message. Finally, the email sent via the send_mail function
Why does the error occur?
We are initializing form under the if request.method == “POST” condition statement. Using the GET request, our variable form doesn’t get defined.
Local variable Referenced before assignment but it is global
This is a common error that happens when we don’t provide a value to a variable and reference it. This can happen with local variables. Global variables can’t be assigned.
This error message is raised when a variable is referenced before it has been assigned a value within the local scope of a function, even though it is a global variable.
Here’s an example to help illustrate the problem:
In this example, x is a global variable that is defined outside of the function my_func(). However, when we try to print the value of x inside the function, we get a UnboundLocalError with the message “local variable ‘x’ referenced before assignment”.
This is because the += operator implicitly creates a local variable within the function’s scope, which shadows the global variable of the same name. Since we’re trying to access the value of x before it’s been assigned a value within the local scope, the interpreter raises an error.
To fix this, you can use the global keyword to explicitly refer to the global variable within the function’s scope:
However, in the above example, the global keyword tells Python that we want to modify the value of the global variable x, rather than creating a new local variable. This allows us to access and modify the global variable within the function’s scope, without causing any errors.
Local variable ‘version’ referenced before assignment ubuntu-drivers
This error occurs with Ubuntu version drivers. To solve this error, you can re-specify the version information and give a split as 2 –
Here, p_name means package name.
With the help of the threading module, you can avoid using global variables in multi-threading. Make sure you lock and release your threads correctly to avoid the race condition.
When a variable that is created locally is called before assigning, it results in Unbound Local Error in Python. The interpreter can’t track the variable.
Therefore, we have examined the local variable referenced before the assignment Exception in Python. The differences between a local and global variable declaration have been explained, and multiple solutions regarding the issue have been provided.
Trending Python Articles
![local variable 'img_gt' referenced before assignment [Fixed] nameerror: name Unicode is not defined](https://www.pythonpool.com/wp-content/uploads/2024/01/Fixed-nameerror-name-Unicode-is-not-defined-300x157.webp)
- Python Course
- Python Basics
- Interview Questions
- Python Quiz
- Popular Packages
- Python Projects
- Practice Python
- AI With Python
- Learn Python3
- Python Automation
- Python Web Dev
- DSA with Python
- Python OOPs
- Dictionaries
How to Fix – UnboundLocalError: Local variable Referenced Before Assignment in Python
Developers often encounter the UnboundLocalError Local Variable Referenced Before Assignment error in Python. In this article, we will see what is local variable referenced before assignment error in Python and how to fix it by using different approaches.
What is UnboundLocalError: Local variable Referenced Before Assignment?
This error occurs when a local variable is referenced before it has been assigned a value within a function or method. This error typically surfaces when utilizing try-except blocks to handle exceptions, creating a puzzle for developers trying to comprehend its origins and find a solution.
Below, are the reasons by which UnboundLocalError: Local variable Referenced Before Assignment error occurs in Python :
Nested Function Variable Access
Global variable modification.
In this code, the outer_function defines a variable ‘x’ and a nested inner_function attempts to access it, but encounters an UnboundLocalError due to a local ‘x’ being defined later in the inner_function.
In this code, the function example_function tries to increment the global variable ‘x’, but encounters an UnboundLocalError since it’s treated as a local variable due to the assignment operation within the function.
Solution for Local variable Referenced Before Assignment in Python
Below, are the approaches to solve “Local variable Referenced Before Assignment”.
In this code, example_function successfully modifies the global variable ‘x’ by declaring it as global within the function, incrementing its value by 1, and then printing the updated value.
In this code, the outer_function defines a local variable ‘x’, and the inner_function accesses and modifies it as a nonlocal variable, allowing changes to the outer function’s scope from within the inner function.
Please Login to comment...
Similar reads.
- Python Programs
- Python Errors
- Python How-to-fix
Improve your Coding Skills with Practice
What kind of Experience do you want to share?
Local variable referenced before assignment in Python
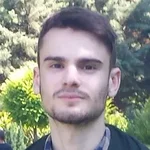
Last updated: Apr 8, 2024 Reading time · 4 min
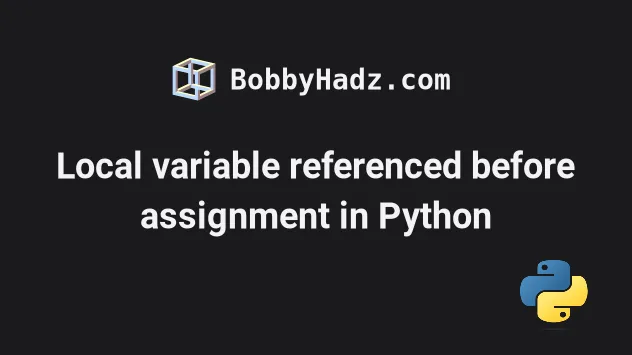
# Local variable referenced before assignment in Python
The Python "UnboundLocalError: Local variable referenced before assignment" occurs when we reference a local variable before assigning a value to it in a function.
To solve the error, mark the variable as global in the function definition, e.g. global my_var .
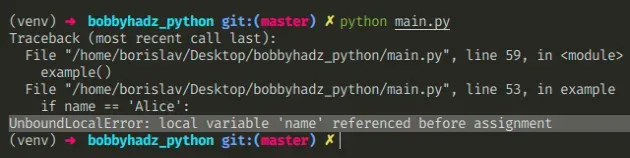
Here is an example of how the error occurs.
We assign a value to the name variable in the function.
# Mark the variable as global to solve the error
To solve the error, mark the variable as global in your function definition.
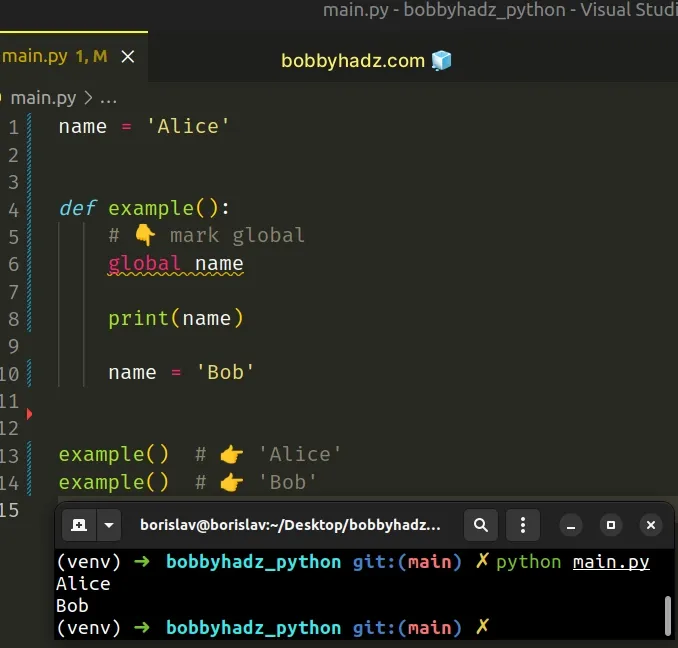
If a variable is assigned a value in a function's body, it is a local variable unless explicitly declared as global .
# Local variables shadow global ones with the same name
You could reference the global name variable from inside the function but if you assign a value to the variable in the function's body, the local variable shadows the global one.
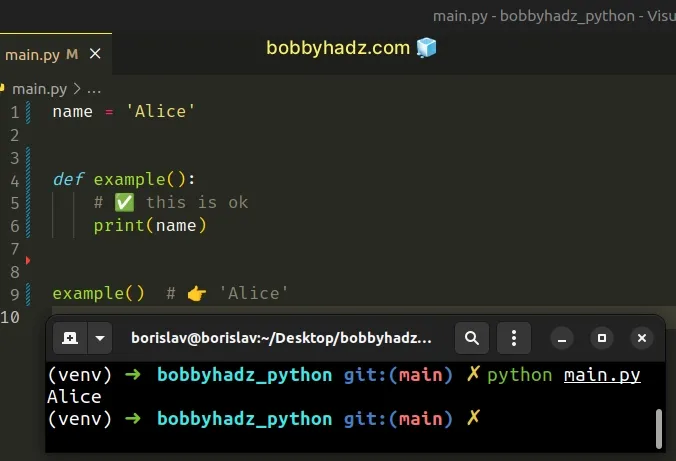
Accessing the name variable in the function is perfectly fine.
On the other hand, variables declared in a function cannot be accessed from the global scope.
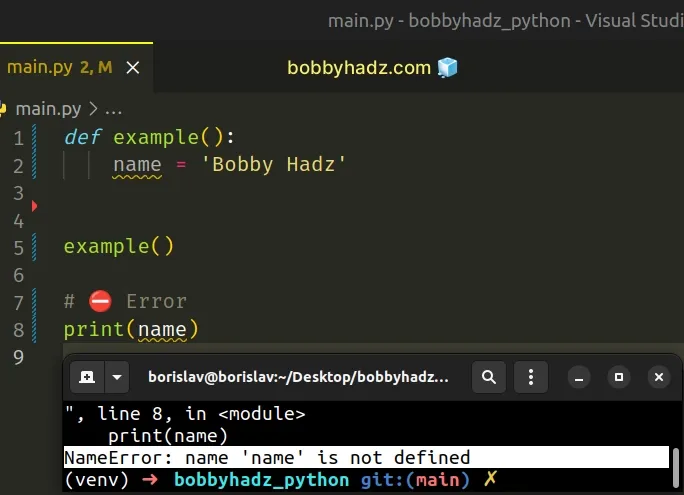
The name variable is declared in the function, so trying to access it from outside causes an error.
Make sure you don't try to access the variable before using the global keyword, otherwise, you'd get the SyntaxError: name 'X' is used prior to global declaration error.
# Returning a value from the function instead
An alternative solution to using the global keyword is to return a value from the function and use the value to reassign the global variable.
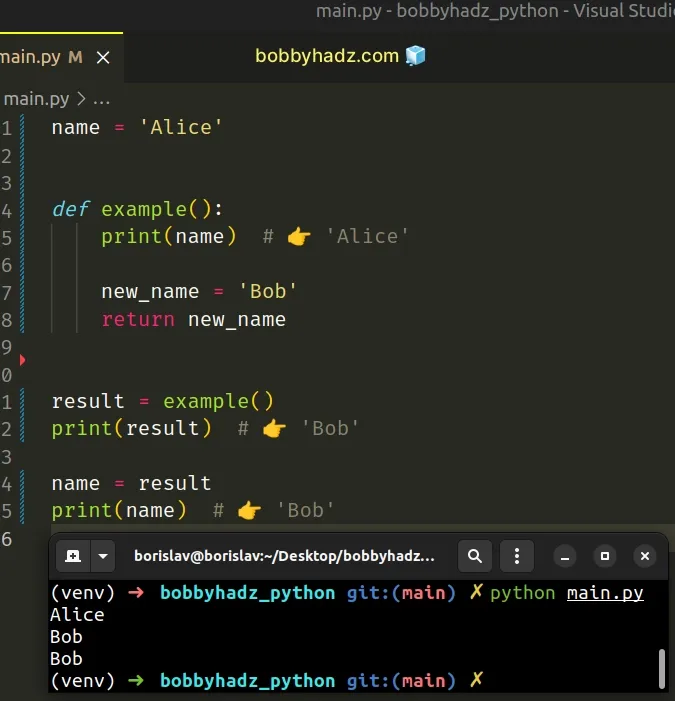
We simply return the value that we eventually use to assign to the name global variable.
# Passing the global variable as an argument to the function
You should also consider passing the global variable as an argument to the function.
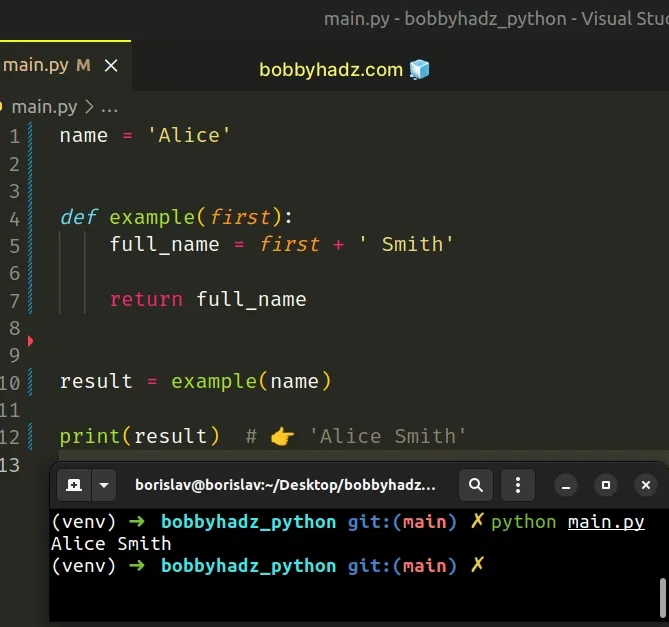
We passed the name global variable as an argument to the function.
If we assign a value to a variable in a function, the variable is assumed to be local unless explicitly declared as global .
# Assigning a value to a local variable from an outer scope
If you have a nested function and are trying to assign a value to the local variables from the outer function, use the nonlocal keyword.
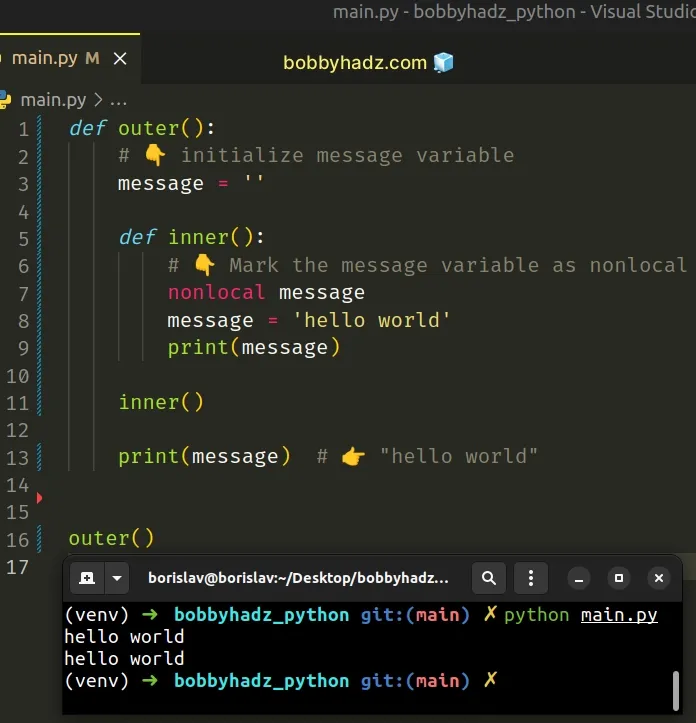
The nonlocal keyword allows us to work with the local variables of enclosing functions.
Had we not used the nonlocal statement, the call to the print() function would have returned an empty string.
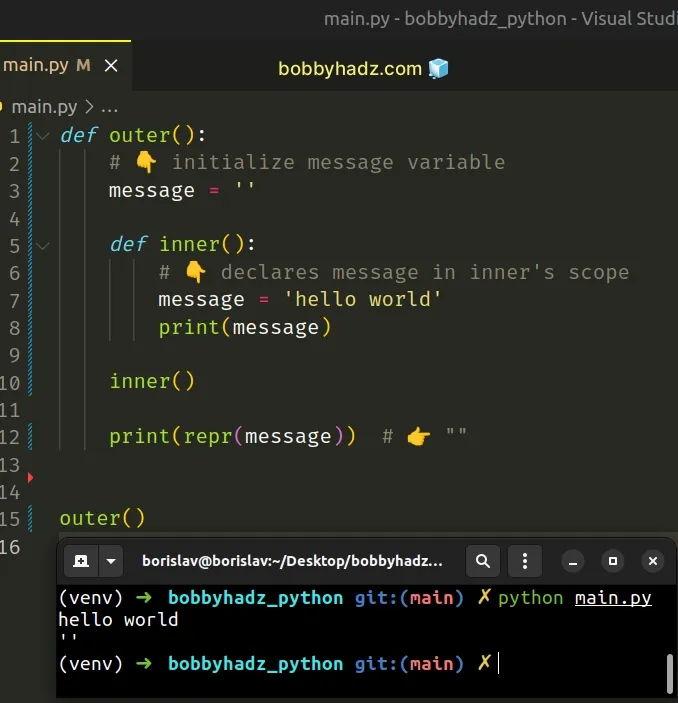
Printing the message variable on the last line of the function shows an empty string because the inner() function has its own scope.
Changing the value of the variable in the inner scope is not possible unless we use the nonlocal keyword.
Instead, the message variable in the inner function simply shadows the variable with the same name from the outer scope.
# Discussion
As shown in this section of the documentation, when you assign a value to a variable inside a function, the variable:
- Becomes local to the scope.
- Shadows any variables from the outer scope that have the same name.
The last line in the example function assigns a value to the name variable, marking it as a local variable and shadowing the name variable from the outer scope.
At the time the print(name) line runs, the name variable is not yet initialized, which causes the error.
The most intuitive way to solve the error is to use the global keyword.
The global keyword is used to indicate to Python that we are actually modifying the value of the name variable from the outer scope.
- If a variable is only referenced inside a function, it is implicitly global.
- If a variable is assigned a value inside a function's body, it is assumed to be local, unless explicitly marked as global .
If you want to read more about why this error occurs, check out [this section] ( this section ) of the docs.
# Additional Resources
You can learn more about the related topics by checking out the following tutorials:
- SyntaxError: name 'X' is used prior to global declaration
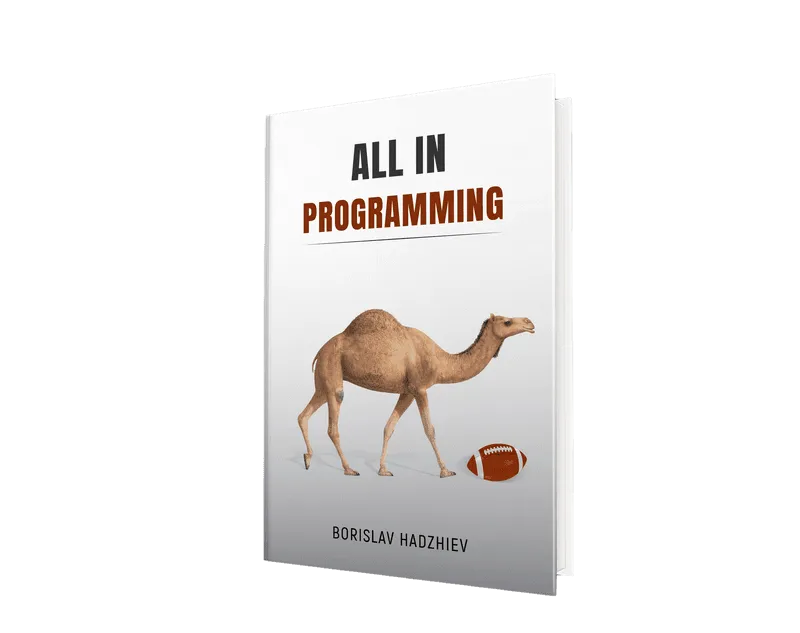
Borislav Hadzhiev
Web Developer
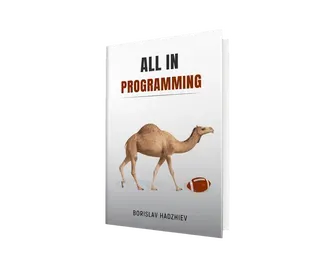
Copyright © 2024 Borislav Hadzhiev
Fix "local variable referenced before assignment" in Python
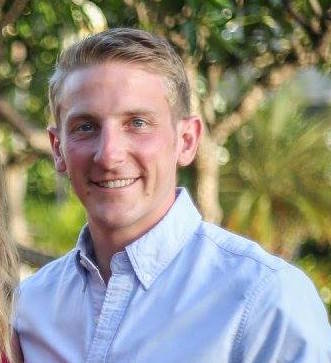
Introduction
If you're a Python developer, you've probably come across a variety of errors, like the "local variable referenced before assignment" error. This error can be a bit puzzling, especially for beginners and when it involves local/global variables.
Today, we'll explain this error, understand why it occurs, and see how you can fix it.
The "local variable referenced before assignment" Error
The "local variable referenced before assignment" error in Python is a common error that occurs when a local variable is referenced before it has been assigned a value. This error is a type of UnboundLocalError , which is raised when a local variable is referenced before it has been assigned in the local scope.
Here's a simple example:
Running this code will throw the "local variable 'x' referenced before assignment" error. This is because the variable x is referenced in the print(x) statement before it is assigned a value in the local scope of the foo function.
Even more confusing is when it involves global variables. For example, the following code also produces the error:
But wait, why does this also produce the error? Isn't x assigned before it's used in the say_hello function? The problem here is that x is a global variable when assigned "Hello ". However, in the say_hello function, it's a different local variable, which has not yet been assigned.
We'll see later in this Byte how you can fix these cases as well.
Fixing the Error: Initialization
One way to fix this error is to initialize the variable before using it. This ensures that the variable exists in the local scope before it is referenced.
Let's correct the error from our first example:
In this revised code, we initialize x with a value of 1 before printing it. Now, when you run the function, it will print 1 without any errors.
Fixing the Error: Global Keyword
Another way to fix this error, depending on your specific scenario, is by using the global keyword. This is especially useful when you want to use a global variable inside a function.
No spam ever. Unsubscribe anytime. Read our Privacy Policy.
Here's how:
In this snippet, we declare x as a global variable inside the function foo . This tells Python to look for x in the global scope, not the local one . Now, when you run the function, it will increment the global x by 1 and print 1 .

Similar Error: NameError
An error that's similar to the "local variable referenced before assignment" error is the NameError . This is raised when you try to use a variable or a function name that has not been defined yet.
Running this code will result in a NameError :
In this case, we're trying to print the value of y , but y has not been defined anywhere in the code. Hence, Python raises a NameError . This is similar in that we are trying to use an uninitialized/undefined variable, but the main difference is that we didn't try to initialize y anywhere else in our code.
Variable Scope in Python
Understanding the concept of variable scope can help avoid many common errors in Python, including the main error of interest in this Byte. But what exactly is variable scope?
In Python, variables have two types of scope - global and local. A variable declared inside a function is known as a local variable, while a variable declared outside a function is a global variable.
Consider this example:
In this code, x is a global variable, and y is a local variable. x can be accessed anywhere in the code, but y can only be accessed within my_function . Confusion surrounding this is one of the most common causes for the "variable referenced before assignment" error.
In this Byte, we've taken a look at the "local variable referenced before assignment" error and another similar error, NameError . We also delved into the concept of variable scope in Python, which is an important concept to understand to avoid these errors. If you're seeing one of these errors, check the scope of your variables and make sure they're being assigned before they're being used.
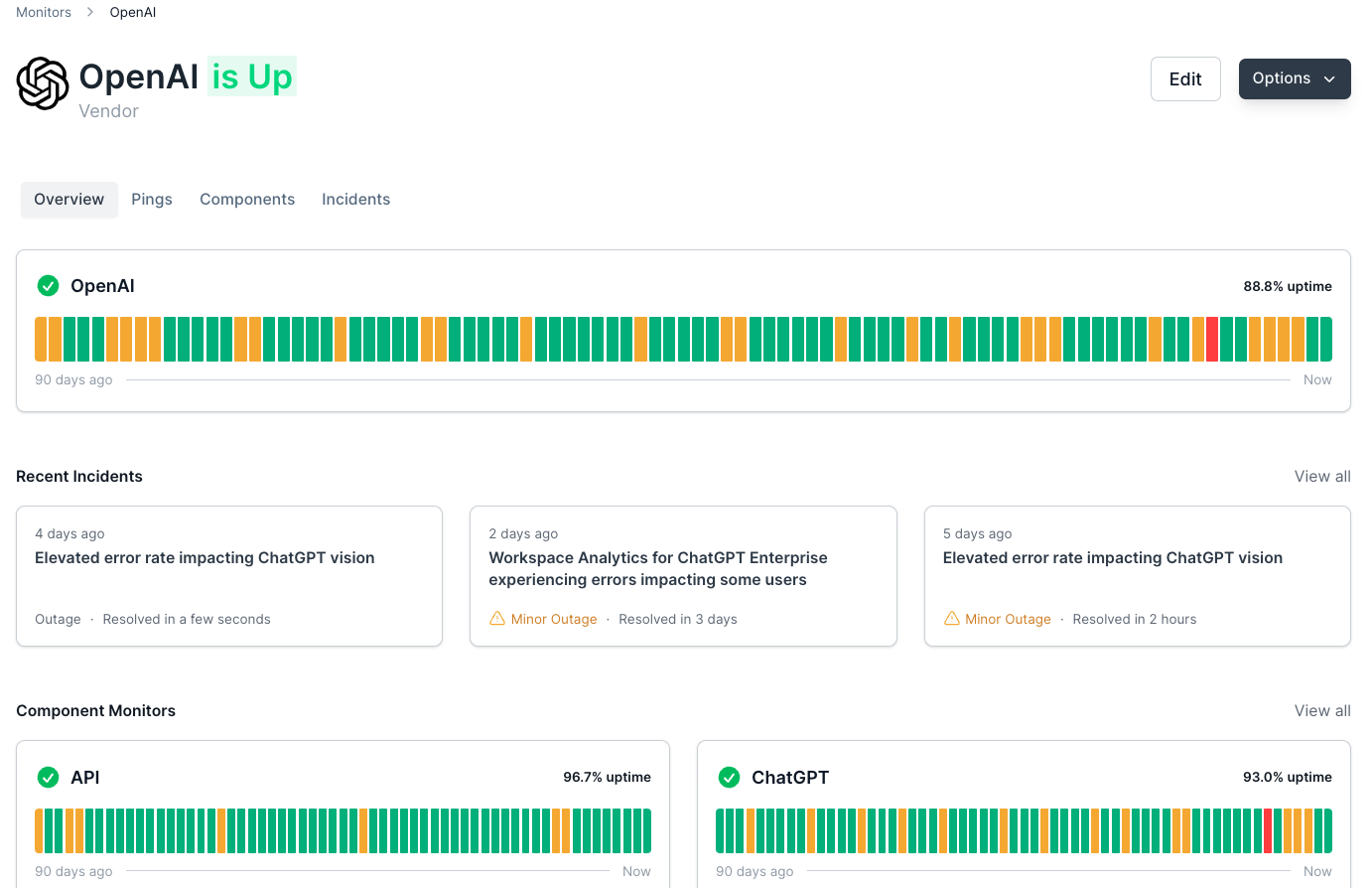
Monitor with Ping Bot
Reliable monitoring for your app, databases, infrastructure, and the vendors they rely on. Ping Bot is a powerful uptime and performance monitoring tool that helps notify you and resolve issues before they affect your customers.
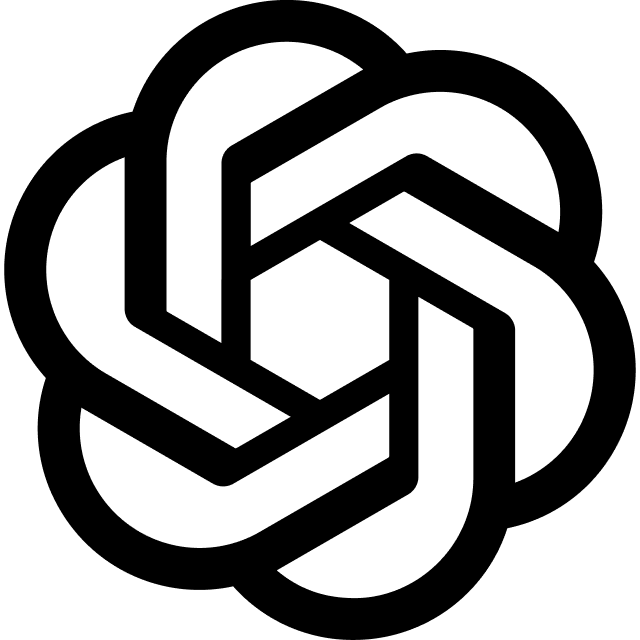
© 2013- 2024 Stack Abuse. All rights reserved.
Explore your training options in 10 minutes Get Started
- Graduate Stories
- Partner Spotlights
- Bootcamp Prep
- Bootcamp Admissions
- University Bootcamps
- Coding Tools
- Software Engineering
- Web Development
- Data Science
- Tech Guides
- Tech Resources
- Career Advice
- Online Learning
- Internships
- Apprenticeships
- Tech Salaries
- Associate Degree
- Bachelor's Degree
- Master's Degree
- University Admissions
- Best Schools
- Certifications
- Bootcamp Financing
- Higher Ed Financing
- Scholarships
- Financial Aid
- Best Coding Bootcamps
- Best Online Bootcamps
- Best Web Design Bootcamps
- Best Data Science Bootcamps
- Best Technology Sales Bootcamps
- Best Data Analytics Bootcamps
- Best Cybersecurity Bootcamps
- Best Digital Marketing Bootcamps
- Los Angeles
- San Francisco
- Browse All Locations
- Digital Marketing
- Machine Learning
- See All Subjects
- Bootcamps 101
- Full-Stack Development
- Career Changes
- View all Career Discussions
- Mobile App Development
- Cybersecurity
- Product Management
- UX/UI Design
- What is a Coding Bootcamp?
- Are Coding Bootcamps Worth It?
- How to Choose a Coding Bootcamp
- Best Online Coding Bootcamps and Courses
- Best Free Bootcamps and Coding Training
- Coding Bootcamp vs. Community College
- Coding Bootcamp vs. Self-Learning
- Bootcamps vs. Certifications: Compared
- What Is a Coding Bootcamp Job Guarantee?
- How to Pay for Coding Bootcamp
- Ultimate Guide to Coding Bootcamp Loans
- Best Coding Bootcamp Scholarships and Grants
- Education Stipends for Coding Bootcamps
- Get Your Coding Bootcamp Sponsored by Your Employer
- GI Bill and Coding Bootcamps
- Tech Intevriews
- Our Enterprise Solution
- Connect With Us
- Publication
- Reskill America
- Partner With Us
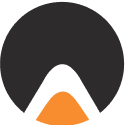
- Resource Center
- Bachelor’s Degree
- Master’s Degree
Python local variable referenced before assignment Solution
When you start introducing functions into your code, you’re bound to encounter an UnboundLocalError at some point. This error is raised when you try to use a variable before it has been assigned in the local context .
In this guide, we talk about what this error means and why it is raised. We walk through an example of this error in action to help you understand how you can solve it.
Find your bootcamp match
What is unboundlocalerror: local variable referenced before assignment.
Trying to assign a value to a variable that does not have local scope can result in this error:
Python has a simple rule to determine the scope of a variable. If a variable is assigned in a function , that variable is local. This is because it is assumed that when you define a variable inside a function you only need to access it inside that function.
There are two variable scopes in Python: local and global. Global variables are accessible throughout an entire program; local variables are only accessible within the function in which they are originally defined.
Let’s take a look at how to solve this error.
An Example Scenario
We’re going to write a program that calculates the grade a student has earned in class.
We start by declaring two variables:
These variables store the numerical and letter grades a student has earned, respectively. By default, the value of “letter” is “F”. Next, we write a function that calculates a student’s letter grade based on their numerical grade using an “if” statement :
Finally, we call our function:
This line of code prints out the value returned by the calculate_grade() function to the console. We pass through one parameter into our function: numerical. This is the numerical value of the grade a student has earned.
Let’s run our code and see what happens:
An error has been raised.
The Solution
Our code returns an error because we reference “letter” before we assign it.
We have set the value of “numerical” to 42. Our if statement does not set a value for any grade over 50. This means that when we call our calculate_grade() function, our return statement does not know the value to which we are referring.
We do define “letter” at the start of our program. However, we define it in the global context. Python treats “return letter” as trying to return a local variable called “letter”, not a global variable.
We solve this problem in two ways. First, we can add an else statement to our code. This ensures we declare “letter” before we try to return it:
Let’s try to run our code again:
Our code successfully prints out the student’s grade.
If you are using an “if” statement where you declare a variable, you should make sure there is an “else” statement in place. This will make sure that even if none of your if statements evaluate to True, you can still set a value for the variable with which you are going to work.
Alternatively, we could use the “global” keyword to make our global keyword available in the local context in our calculate_grade() function. However, this approach is likely to lead to more confusing code and other issues. In general, variables should not be declared using “global” unless absolutely necessary . Your first, and main, port of call should always be to make sure that a variable is correctly defined.
In the example above, for instance, we did not check that the variable “letter” was defined in all use cases.
That’s it! We have fixed the local variable error in our code.
The UnboundLocalError: local variable referenced before assignment error is raised when you try to assign a value to a local variable before it has been declared. You can solve this error by ensuring that a local variable is declared before you assign it a value.
Now you’re ready to solve UnboundLocalError Python errors like a professional developer !
About us: Career Karma is a platform designed to help job seekers find, research, and connect with job training programs to advance their careers. Learn about the CK publication .
What's Next?

Get matched with top bootcamps
Ask a question to our community, take our careers quiz.
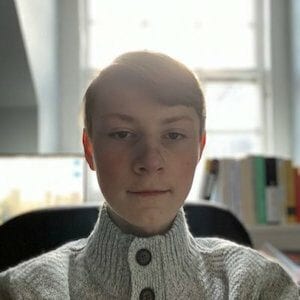
Leave a Reply Cancel reply
Your email address will not be published. Required fields are marked *
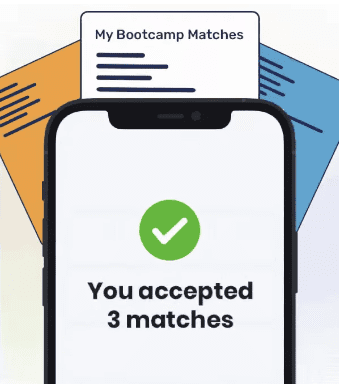
How to Fix Local Variable Referenced Before Assignment Error in Python
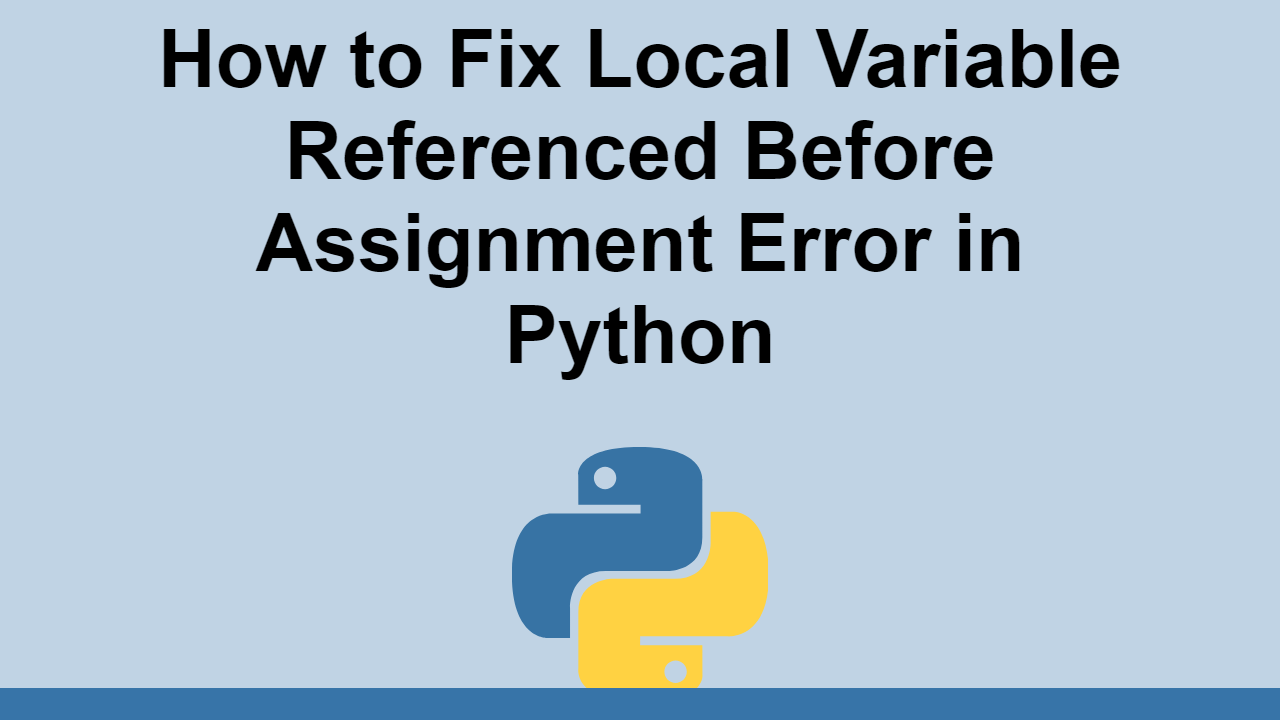
Table of Contents
Fixing local variable referenced before assignment error.
In Python , when you try to reference a variable that hasn't yet been given a value (assigned), it will throw an error.
That error will look like this:
In this post, we'll see examples of what causes this and how to fix it.
Let's begin by looking at an example of this error:
If you run this code, you'll get
The issue is that in this line:
We are defining a local variable called value and then trying to use it before it has been assigned a value, instead of using the variable that we defined in the first line.
If we want to refer the variable that was defined in the first line, we can make use of the global keyword.
The global keyword is used to refer to a variable that is defined outside of a function.
Let's look at how using global can fix our issue here:
Global variables have global scope, so you can referenced them anywhere in your code, thus avoiding the error.
If you run this code, you'll get this output:
In this post, we learned at how to avoid the local variable referenced before assignment error in Python.
The error stems from trying to refer to a variable without an assigned value, so either make use of a global variable using the global keyword, or assign the variable a value before using it.
Thanks for reading!
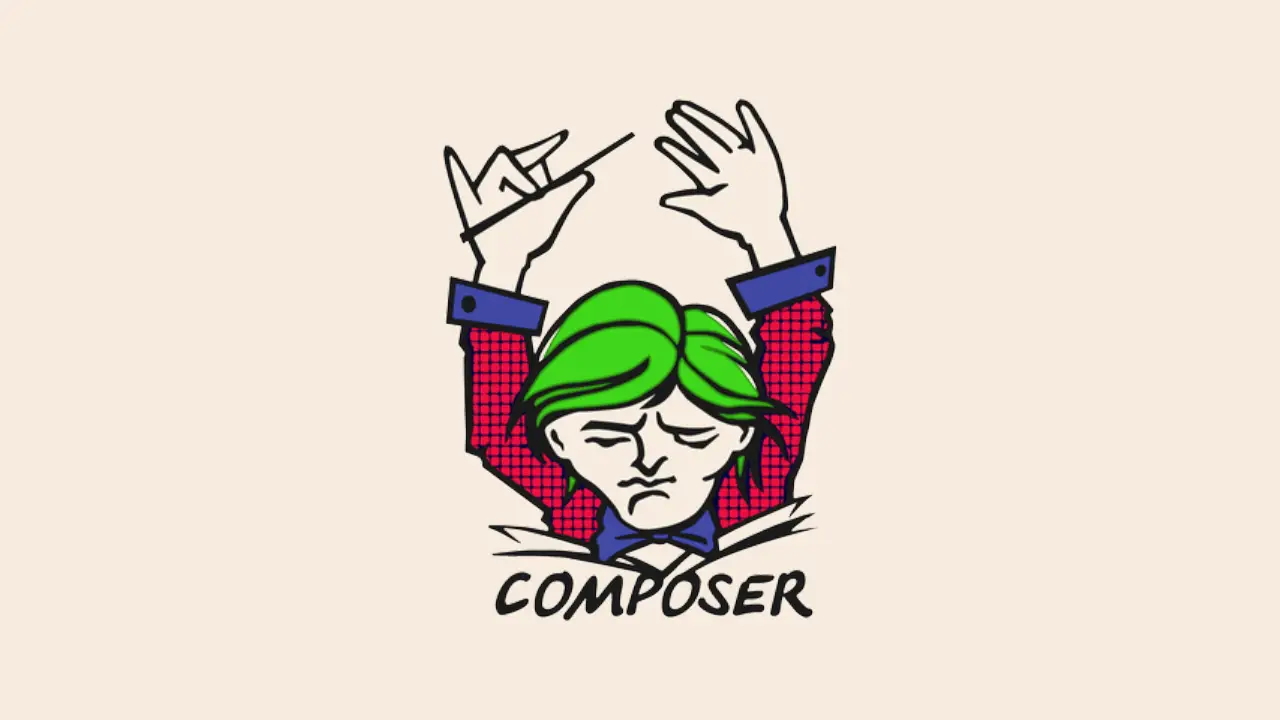
- Privacy Policy
- Terms of Service
Adventures in Machine Learning
4 ways to fix local variable referenced before assignment error in python, resolving the local variable referenced before assignment error in python.
Python is one of the world’s most popular programming languages due to its simplicity, readability, and versatility. Despite its many advantages, when coding in Python, one may encounter various errors, with the most common being the “local variable referenced before assignment” error.
Even the most experienced Python developers have encountered this error at some point in their programming career. In this article, we will look at four effective strategies for resolving the local variable referenced before assignment error in Python.
Strategy 1: Assigning a Value before Referencing
The first strategy is to assign a value to a variable before referencing it. The error occurs when the variable is referenced before it is assigned a value.
This problem can be avoided by initializing the variable before referencing it. For example, let us consider the snippet below:
In the snippet above, the variables x and y are not assigned values before they are referenced in the print statement. Therefore, we will get a local variable “referenced before assignment” error.
To resolve this error, we must initialize the variables before referencing them. We can avoid this error by assigning a value to x and y before they are referenced, as shown below:
Strategy 2: Using the Global Keyword
In Python, variables declared inside a function are considered local variables. Thus, they are separate from other variables declared outside of the function.
If we want to use a variable outside of the function, we must use the global keyword. Using the global keyword tells Python that you want to use the variable that was defined globally, not locally.
For example:
In the code snippet above, the global keyword tells Python to use the variable x defined outside of the function rather than a local variable named x . Thus, Python will output 30.
Strategy 3: Adding Input Parameters for Functions
Another way to avoid the local variable referenced before assignment error is by adding input parameters to functions.
In the code snippet above, x and y are variables that are passed into the add_numbers function as arguments.
This approach allows us to avoid the local variable referenced before assignment error because the variables are being passed into the function as input parameters.
Strategy 4: Initializing Variables before Loops or Conditionals
Finally, it’s also a good practice to initialize the variables before loops or conditionals.
If you are defining a variable within a loop, you must initialize it before the loop starts. This way, the variable already exists, and we can update the value inside the loop.
In the code snippet above, the variable sum has been initialized with the value of 0 before the loop runs. Thus, we can update and use the variable inside the loop.
In conclusion, the “local variable referenced before assignment” error is a common issue in Python. However, with the strategies discussed in this article, you can avoid the error and write clean Python code.
Remember to initialize your variables, use the global keyword, add input parameters in functions, and initialize variables before loops or conditionals. By following these techniques, your Python code will be error-free and much easier to manage.
In essence, this article has provided four key strategies for resolving the “local variable referenced before assignment” error that is common in Python. These strategies include initializing variables before referencing, using the global keyword, adding input parameters to functions, and initializing variables before loops or conditionals.
These techniques help to ensure clean code that is free from errors. By implementing these strategies, developers can improve their code quality and avoid time-wasting errors that can occur in their work.
Popular Posts
Efficient data management: exporting and importing mysql data with csv files, mastering the sql and operator: examples and usage, mastering precision and recall metrics in machine learning.
- Terms & Conditions
- Privacy Policy
How to Solve Error - Local Variable Referenced Before Assignment in Python
- Python How-To's
- How to Solve Error - Local Variable …
Check the Variable Scope to Fix the local variable referenced before assignment Error in Python
Initialize the variable before use to fix the local variable referenced before assignment error in python, use conditional assignment to fix the local variable referenced before assignment error in python.
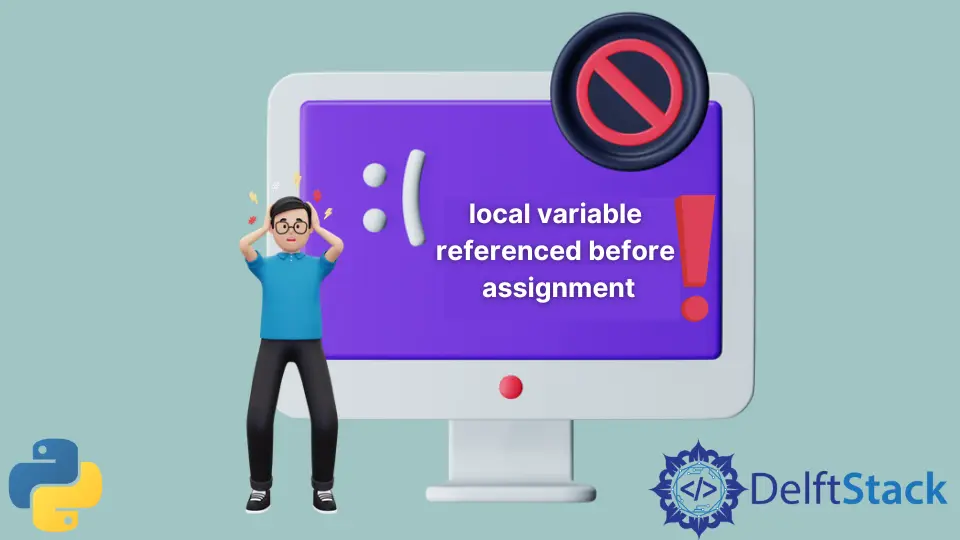
This article delves into various strategies to resolve the common local variable referenced before assignment error. By exploring methods such as checking variable scope, initializing variables before use, conditional assignments, and more, we aim to equip both novice and seasoned programmers with practical solutions.
Each method is dissected with examples, demonstrating how subtle changes in code can prevent this frequent error, enhancing the robustness and readability of your Python projects.
The local variable referenced before assignment occurs when some variable is referenced before assignment within a function’s body. The error usually occurs when the code is trying to access the global variable.
The primary purpose of managing variable scope is to ensure that variables are accessible where they are needed while maintaining code modularity and preventing unexpected modifications to global variables.
We can declare the variable as global using the global keyword in Python. Once the variable is declared global, the program can access the variable within a function, and no error will occur.
The below example code demonstrates the code scenario where the program will end up with the local variable referenced before assignment error.
In this example, my_var is a global variable. Inside update_var , we attempt to modify it without declaring its scope, leading to the Local Variable Referenced Before Assignment error.
We need to declare the my_var variable as global using the global keyword to resolve this error. The below example code demonstrates how the error can be resolved using the global keyword in the above code scenario.
In the corrected code, we use the global keyword to inform Python that my_var references the global variable.
When we first print my_var , it displays the original value from the global scope.
After assigning a new value to my_var , it updates the global variable, not a local one. This way, we effectively tell Python the scope of our variable, thus avoiding any conflicts between local and global variables with the same name.
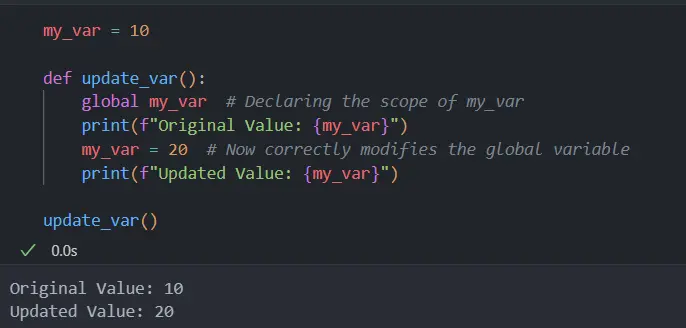
Ensure that the variable is initialized with some value before using it. This can be done by assigning a default value to the variable at the beginning of the function or code block.
The main purpose of initializing variables before use is to ensure that they have a defined state before any operations are performed on them. This practice is not only crucial for avoiding the aforementioned error but also promotes writing clear and predictable code, which is essential in both simple scripts and complex applications.
In this example, the variable total is used in the function calculate_total without prior initialization, leading to the Local Variable Referenced Before Assignment error. The below example code demonstrates how the error can be resolved in the above code scenario.
In our corrected code, we initialize the variable total with 0 before using it in the loop. This ensures that when we start adding item values to total , it already has a defined state (in this case, 0).
This initialization is crucial because it provides a starting point for accumulation within the loop. Without this step, Python does not know the initial state of total , leading to the error.
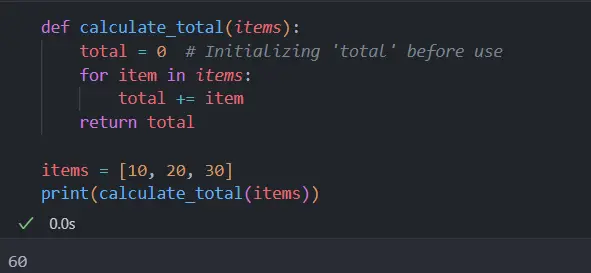
Conditional assignment allows variables to be assigned values based on certain conditions or logical expressions. This method is particularly useful when a variable’s value depends on certain prerequisites or states, ensuring that a variable is always initialized before it’s used, thereby avoiding the common error.
In this example, message is only assigned within the if and elif blocks. If neither condition is met (as with guest ), the variable message remains uninitialized, leading to the Local Variable Referenced Before Assignment error when trying to print it.
The below example code demonstrates how the error can be resolved in the above code scenario.
In the revised code, we’ve included an else statement as part of our conditional logic. This guarantees that no matter what value user_type holds, the variable message will be assigned some value before it is used in the print function.
This conditional assignment ensures that the message is always initialized, thereby eliminating the possibility of encountering the Local Variable Referenced Before Assignment error.
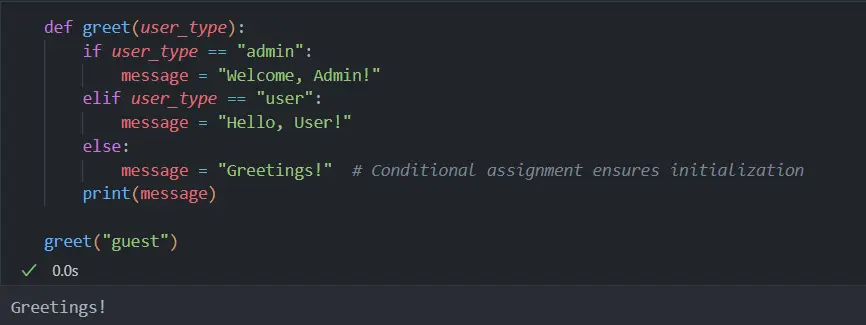
Throughout this article, we have explored multiple approaches to address the Local Variable Referenced Before Assignment error in Python. From the nuances of variable scope to the effectiveness of initializations and conditional assignments, these strategies are instrumental in developing error-free code.
The key takeaway is the importance of understanding variable scope and initialization in Python. By applying these methods appropriately, programmers can not only resolve this specific error but also enhance the overall quality and maintainability of their code, making their programming journey smoother and more rewarding.
【Python】成功解决UnboundLocalError: local variable ‘a‘ referenced before assignment(几种场景下的解决方案)

【Python】成功解决UnboundLocalError: local variable ‘a’ referenced before assignment(几种场景下的解决方案)
🌈 欢迎莅临 我的 个人主页 👈这里是我 静心耕耘 深度学习领域、 真诚分享 知识与智慧的小天地!🎇 🎓 博主简介 : 985高校 的普通本硕,曾有幸发表过人工智能领域的 中科院顶刊一作论文,熟练掌握PyTorch框架 。 🔧 技术专长 : 在 CV 、 NLP 及 多模态 等领域有丰富的项目实战经验。已累计 一对一 为数百位用户提供 近千次 专业服务,助力他们 少走弯路、提高效率,近一年 好评率100% 。 📝 博客风采 : 积极分享关于 深度学习、PyTorch、Python 相关的实用内容。已发表原创文章 500余篇 ,代码分享次数 逾四万次 。 💡 服务项目 :包括但不限于 科研入门辅导 、 知识付费答疑 以及 个性化需求解决 。
欢迎添加👉👉👉 底部微信(gsxg605888) 👈👈👈与我交流 ( 请您备注来意 ) ( 请您备注来意 ) ( 请您备注来意 )
🌵文章目录🌵
🐛一、什么是unboundlocalerror?, 🛠️二、如何解决unboundlocalerror?, 🌐三、实际场景中的解决方案, 📖四、深入理解作用域与变量生命周期, 🔍五、举一反三:其他常见错误与陷阱, 💡六、总结与最佳实践, 🎉结语.
在Python编程中, UnboundLocalError: local variable 'a' referenced before assignment 这个错误常常让初学者感到困惑。这个错误表明 你尝试在一个函数内部引用了一个局部变量,但是在引用之前并没有对它进行赋值 。换句话说, Python解释器在函数的作用域内找到了一个变量的引用,但是这个变量并没有在引用它之前被定义或赋值 。
下面是一个简单的例子,演示了如何触发这个错误:
在这个例子中,我们尝试在 a 被赋值之前就打印它的值,这会导致 UnboundLocalError 。
要解决 UnboundLocalError ,你需要 确保在引用局部变量之前,该变量已经被正确地赋值 。这可以通过几种不同的方式实现。
确保在引用局部变量之前,该变量已经被正确赋值。
如果你打算在函数内部引用的是全局变量,那么需要使用 global 关键字来明确指定。
如果你希望变量有一个默认值,你可以使用函数的参数来提供这个默认值。
在某些情况下,你可能需要在使用变量之前检查它是否已经被定义。这可以通过使用 try-except 块来实现。
在实际编程中, UnboundLocalError 可能会出现在更复杂的场景中。下面是一些实际案例及其解决方案。
场景1:在循环中引用和修改变量
在正确示例中,我们在循环中累加 i 到 total ,并在循环结束后打印 total 。注意,我们在累加之前已经对 total 进行了初始化,避免了 UnboundLocalError 。
场景2:在条件语句中引用变量
在正确示例中,我们在 if 语句中根据 x 的值计算 y ,然后在 if 语句外部打印 y 的值。我们使用了 if-else 语句确保了 y 在引用之前一定会被定义。
在解决 UnboundLocalError 时,理解Python中的作用域和变量生命周期至关重要。作用域决定了变量的可见性,即变量在哪里可以被访问。而变量的生命周期则关系到变量的创建和销毁的时机。局部变量只在函数内部可见,并且当函数执行完毕后,它们的生命周期就结束了。全局变量在整个程序中都是可见的,它们的生命周期则与程序的生命周期一致。
除了 UnboundLocalError 之外,Python编程中还有其他一些与变量作用域和生命周期相关的常见错误和陷阱。例如,不小心修改了全局变量而没有意识到,或者在循环中意外地创建了一个新的变量而不是更新现有的变量。避免这些错误的关键在于保持对变量作用域和生命周期的清晰理解,并谨慎地使用 global 关键字。
解决 UnboundLocalError 的关键在于确保在引用局部变量之前已经对其进行了赋值。这可以通过在引用前赋值、使用全局变量、使用默认值或检查变量是否已定义等方式实现。同时,深入理解作用域和变量生命周期对于避免此类错误至关重要。最佳实践包括:
- 在函数内部使用局部变量时,确保在引用之前已经对其进行了赋值。
- 如果需要在函数内部修改全局变量,请使用 global 关键字明确声明。
- 尽量避免在函数内部意外地创建新的全局变量。
- 对于复杂的逻辑,使用明确的变量命名和注释来提高代码的可读性和可维护性。
通过遵循这些最佳实践,你可以减少 UnboundLocalError 的发生,并编写出更加健壮和可靠的Python代码。
通过本文的学习,相信你已经对 UnboundLocalError 有了更深入的理解,并掌握了解决这一错误的几种方法。在实际编程中,遇到问题时不要害怕,要勇于探索和实践。通过不断学习和积累经验,你会逐渐成为一名优秀的Python程序员。加油!🚀
🌈 个人主页:高斯小哥 🔥 高质量专栏:Matplotlib之旅:零基础精通数据可视化 、 Python基础【高质量合集】 、 PyTorch零基础入门教程 👈 希望得到您的订阅和支持~ 💡 创作高质量博文(平均质量分92+),分享更多关于深度学习、PyTorch、Python领域的优质内容!(希望得到您的关注~)
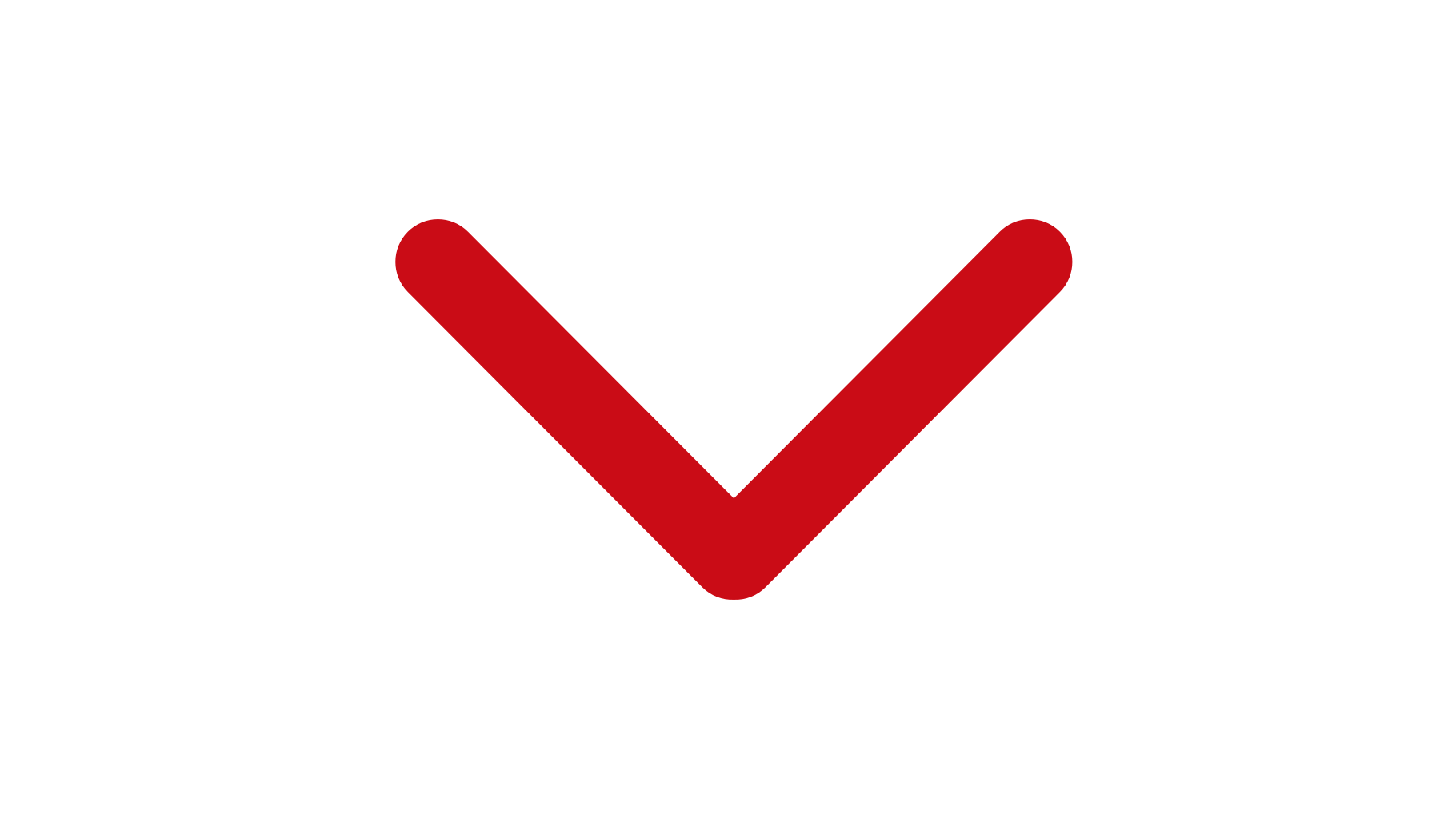
请填写红包祝福语或标题
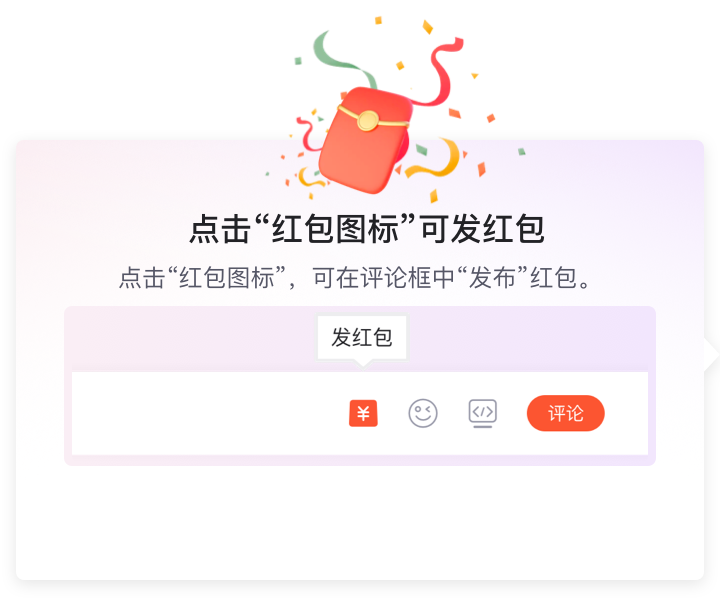
你的鼓励将是我创作的最大动力

您的余额不足,请更换扫码支付或 充值

1.余额是钱包充值的虚拟货币,按照1:1的比例进行支付金额的抵扣。 2.余额无法直接购买下载,可以购买VIP、付费专栏及课程。

Navigation Menu
Search code, repositories, users, issues, pull requests..., provide feedback.
We read every piece of feedback, and take your input very seriously.
Saved searches
Use saved searches to filter your results more quickly.
To see all available qualifiers, see our documentation .
- Notifications You must be signed in to change notification settings
Have a question about this project? Sign up for a free GitHub account to open an issue and contact its maintainers and the community.
By clicking “Sign up for GitHub”, you agree to our terms of service and privacy statement . We’ll occasionally send you account related emails.
Already on GitHub? Sign in to your account
UnboundLocalError: local variable'img_bytes' referenced before assignment #278
shua1z commented Mar 13, 2022
it happened when reading gt pictures , did that means i had a wrong format of pictures such as png-GRAY8BPP ,which isnt fit the codes ? |
The text was updated successfully, but these errors were encountered: |
i have checked the path to pics is right but it cannot load in |
Sorry, something went wrong.
No branches or pull requests
質問をすることでしか得られない、回答やアドバイスがある。
15分調べてもわからないことは、質問しよう!.
Pythonは、コードの読みやすさが特徴的なプログラミング言語の1つです。 強い型付け、動的型付けに対応しており、後方互換性がないバージョン2系とバージョン3系が使用されています。 商用製品の開発にも無料で使用でき、OSだけでなく仮想環境にも対応。Unicodeによる文字列操作をサポートしているため、日本語処理も標準で可能です。
エラー「local variable 'files' referenced before assignment」

投稿 2018/10/01 04:30
PSNRを使って超解像の評価をしたいのですが、うまく動きません。現在でているエラーが「local variable 'files' referenced before assignment」というエラーです。解決法がわかる方がいましたら、ご教示ください。以下が実装コードです。
気になる質問をクリップする
クリップした質問は、後からいつでもMYページで確認できます。
またクリップした質問に回答があった際、通知やメールを受け取ることができます。
バッドをするには、ログインかつ
こちら の条件を満たす必要があります。

2018/10/01 04:36
2018/10/01 04:39
2018/10/01 06:24

local variable 'files' referenced before assignment
日本語に訳すと「 files というローカル変数が代入される前に参照されています」という内容です。
この箇所が for root, dirs, files in os.walk(inputpath): のスコープ外にあるので、 files は利用できません。
投稿 2018/10/01 06:35
2018/10/01 06:52
2018/10/01 07:06
2018/10/01 07:28
PIL を使うと、画像ファイルの内容に応じて、フォーマットのまま読み込めます。
あと PSNR の計算式が間違っていると思います。
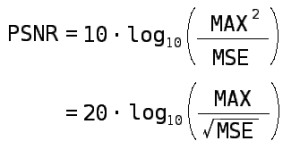
投稿 2018/10/01 09:48

総合スコア 21956
2018/10/01 23:51
質問の解決につながる回答をしましょう。 サンプルコードなど、より具体的な説明があると質問者の理解の助けになります。 また、読む側のことを考えた、分かりやすい文章を心がけましょう。
15分調べてもわからないことは teratailで質問しよう!
ただいまの回答率 85 . 40 %
質問をまとめることで 思考を整理して素早く解決
テンプレート機能で 簡単に質問をまとめる
- Stack Overflow for Teams Where developers & technologists share private knowledge with coworkers
- Advertising & Talent Reach devs & technologists worldwide about your product, service or employer brand
- OverflowAI GenAI features for Teams
- OverflowAPI Train & fine-tune LLMs
- Labs The future of collective knowledge sharing
- About the company Visit the blog
Collectives™ on Stack Overflow
Find centralized, trusted content and collaborate around the technologies you use most.
Q&A for work
Connect and share knowledge within a single location that is structured and easy to search.
Get early access and see previews of new features.
UnboundLocalError: local variable 'img_files' referenced before assignment
I am using python 3.6.0b2 on Windows 64-bit. Below is a python code which trains a keras model with images.
When I run the above code I get an error. The trace back is given below.

img_files will only be available in case of if statements are passed otherwise it will not be bound to a value.
if dataset is not DRIVE or STARE then img_files will not have value.

Your Answer
Reminder: Answers generated by artificial intelligence tools are not allowed on Stack Overflow. Learn more
Sign up or log in
Post as a guest.
Required, but never shown
By clicking “Post Your Answer”, you agree to our terms of service and acknowledge you have read our privacy policy .
Not the answer you're looking for? Browse other questions tagged python-3.x keras or ask your own question .
- The Overflow Blog
- Scaling systems to manage all the metadata ABOUT the data
- Navigating cities of code with Norris Numbers
- Featured on Meta
- We've made changes to our Terms of Service & Privacy Policy - July 2024
- Bringing clarity to status tag usage on meta sites
- Tag hover experiment wrap-up and next steps
Hot Network Questions
- How to Vertically Join Images?
- What is a word/phrase that best describes a "blatant disregard or neglect" for something, but with the connotation of that they should have known?
- Inaccurate group pace
- What is the meaning of "Exit, pursued by a bear"?
- Confused about topographic data in base map using QGIS
- Someone wants to pay me to be his texting buddy. How am I being scammed?
- Erase the loops
- Why are extremally disconnected spaces so hard to give examples of?
- Blocking between two MERGE queries inserting into the same table
- What would be the optimal amount of pulses per second for pulsed laser rifles?
- Do space stations have anything that big spacecraft (such as the Space Shuttle and SpaceX Starship) don't have?
- A short story about a demon, in a modernising Japan, living in electric wires and starting fires
- Making blackberry Jam with fully-ripe blackberries
- Why did evolution fail to protect humans against sun?
- Can a Statute of Limitations claim be rejected by the court?
- When is internal use internal (considering licenses and their obligations)?
- Clarification on Counterfactual Outcomes in Causal Inference
- Multiplication of operators defined by commutation relations
- can a CPU once removed retain information that poses a security concern?
- What determines whether an atheist's claim to be a Christian is logically sound?
- Word to classify what powers a god is associated with?
- In "Take [action]. When you do, [effect]", is the action a cost or an instruction?
- Zipping Many Files
- How to let LaTeX ignore a part of a \section{....} argument for writing the .aux file?

IMAGES
COMMENTS
File "weird.py", line 5, in main. print f(3) UnboundLocalError: local variable 'f' referenced before assignment. Python sees the f is used as a local variable in [f for f in [1, 2, 3]], and decides that it is also a local variable in f(3). You could add a global f statement: def f(x): return x. def main():
The line it's pointing to doesn't even reference img. That should be one of the first things you do when debugging. That should be one of the first things you do when debugging. Then you'd want to make a minimal reproducible example .
DJANGO - Local Variable Referenced Before Assignment [Form] The program takes information from a form filled out by a user. Accordingly, an email is sent using the information. ... Therefore, we have examined the local variable referenced before the assignment Exception in Python. The differences between a local and global variable ...
Output. Hangup (SIGHUP) Traceback (most recent call last): File "Solution.py", line 7, in <module> example_function() File "Solution.py", line 4, in example_function x += 1 # Trying to modify global variable 'x' without declaring it as global UnboundLocalError: local variable 'x' referenced before assignment Solution for Local variable Referenced Before Assignment in Python
If a variable is assigned a value in a function's body, it is a local variable unless explicitly declared as global. # Local variables shadow global ones with the same name You could reference the global name variable from inside the function but if you assign a value to the variable in the function's body, the local variable shadows the global one.
Reliable monitoring for your app, databases, infrastructure, and the vendors they rely on. Ping Bot is a powerful uptime and performance monitoring tool that helps notify you and resolve issues before they affect your customers.
Trying to assign a value to a variable that does not have local scope can result in this error: UnboundLocalError: local variable referenced before assignment. Python has a simple rule to determine the scope of a variable. If a variable is assigned in a function, that variable is local. This is because it is assumed that when you define a ...
value = value + 1 print (value) increment() If you run this code, you'll get. BASH. UnboundLocalError: local variable 'value' referenced before assignment. The issue is that in this line: PYTHON. value = value + 1. We are defining a local variable called value and then trying to use it before it has been assigned a value, instead of using the ...
Strategy 2: Using the Global Keyword. In Python, variables declared inside a function are considered local variables. Thus, they are separate from other variables declared outside of the function.
This tutorial explains the reason and solution of the python error local variable referenced before assignment
When you run detection using this code: image_id = random.choice(dataset.image_ids) image, image_meta, gt_class_id, gt_bbox, gt_mask =\ modellib.load_image_gt(dataset ...
ccordoba12 changed the title spyder3: UnboundLocalError: local variable 'img_path' referenced before assignment UnboundLocalError: local variable 'img_path' referenced before assignment Feb 10, 2017. dalthviz mentioned this issue Feb 10, 2017. PR: Add an initialization value to img_path #4133.
local variable referenced before assignment 原因及解决办法. 一句话, 在函数内部更改全局变量就会出现此错误 。. 在上面一段代码中,函数temp的操作是打印a的值,但函数内部并没有对a进行定义,此时系统会在外部寻找a的值,而我们在函数外部给a赋值为3,这种 在函数 ...
Error: UnboundLocalError: local variable 'img' referenced before assignment Reason: I passed the image read using imageio.imread() Solution: Please add an if case for that.
In order for you to modify test1 while inside a function you will need to do define test1 as a global variable, for example: test1 = 0. def test_func(): global test1. test1 += 1. test_func() However, if you only need to read the global variable you can print it without using the keyword global, like so: test1 = 0.
If you want to set `OMP_NUM_THREADS` manually, please export it on the command line before running a python script. e.g. `export OMP_NUM_THREADS=12; python your_program.py`. It is recommended to set it below 24.
在Python编程中, UnboundLocalError: local variable 'a' referenced before assignment 这个错误常常让初学者感到困惑。. 这个错误表明 你尝试在一个函数内部引用了一个局部变量,但是在引用之前并没有对它进行赋值 。. 换句话说, Python解释器在函数的作用域内找到了一个变量 ...
I am glad that deleting your pyc files solved your problem. However, your code doesn't actually call for global variables. In most of the cases the (evitable) use of globals is a sign of not so good design and may cause all sorts of problems, including name clashes etc.
it happened when reading gt pictures , did that means i had a wrong format of pictures such as png-GRAY8BPP ,which isnt fit the codes ? ... local variable'img_bytes' referenced before assignment #278. Closed ... Closed UnboundLocalError: local variable'img_bytes' referenced before assignment #278. shua1z opened this issue Mar 13, 2022 · 1 ...
PSNRを使って超解像の評価をしたいのですが、うまく動きません。現在でているエラーが「local variable 'files' referenced before assignment」というエラーです。解決法がわかる方がいましたら、ご教示ください。以下が実装コードです。
UnboundLocalError: local variable 'img_files' referenced before assignment. Ask Question Asked 5 years, 11 months ago. Modified 5 years, 11 months ago. Viewed 488 times ... \New folder\codes\utils.py", line 314, in get_imgs fundus_imgs=imagefiles2arrs(img_files) UnboundLocalError: local variable 'img_files' referenced before assignment ...